Display Notifications on a Galaxy Watch Running Wear OS Powered by Samsung
Shamima Nasrin
Engineer, Samsung Developer Program
Notifications are a central part of many mobile applications because they keep users updated and engaged. So, implementing them in your application can greatly enhance the user experience.
Creating notifications on Android wearables is very simple, but for security or user experience reasons, manufacturers can have varying policies related to notifications. When developing your application, you must take these policies into account when implementing notifications for the real devices that the application supports.
This tutorial demonstrates how to easily implement notifications on a Galaxy Watch running Wear OS Powered by Samsung and introduces the notification policies specific to Galaxy Watch. A sample application is also provided so you can examine how the code works in practice.
Implementing Notifications
To implement a basic notification for Wear OS Powered by Samsung devices:
-
In Android Studio, to create a wearable application project, select "New Project > Wear OS > Blank Activity > Finish."
-
In the application code, create an instance of the
NotificationManager
class:NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
-
To implement the notification icon, store the icon image as a PNG file within the "res" folder in the project. The following code converts the image into a bitmap:
Drawable drawable = ResourcesCompat.getDrawable(getResources(),R.drawable.notification_icon, null); BitmapDrawable bitmapDrawable = (BitmapDrawable) drawable; Bitmap largeIcon = bitmapDrawable.getBitmap();
-
To define the notification properties, such as the icon, title, and text, use
Notification.Builder
:Notification notification= new Notification.Builder(this) .setLargeIcon(largeIcon) .setSmallIcon(R.drawable.notification_icon) .setContentText(“Hello Samsung!”) .setChannelId(CHANNEL_ID) .build();
-
Create a notification channel and assign it a unique channel ID. This is required for Android 8.0 and higher.
notificationManager.createNotificationChannel(new NotificationChannel(CHANNEL_ID,CHANNEL_NAME, NotificationManager.IMPORTANCE_DEFAULT));
-
Display the notification:
notificationManager.notify(100, notification);
In the sample application, the notification is assigned a random integer value to uniquely identify the notification. You need this notification ID to update or dismiss the notification.
Notification Policies for Galaxy Watch
To implement notifications appropriately for Galaxy Watch, you must understand the following policies related to notification behavior:
-
Notifications, including their sound or vibration, can only be triggered when the watch is being worn on the wrist. To check whether the user is wearing the watch, use the SensorManager library. If the value of the sensor key
TYPE_OFFBODY_DETECTION
is "1," the watch is being worn and notifications can be triggered. -
Since background services consume significant battery power, Samsung restricts background services from third-party applications to balance user experience and battery life. If you want to send notifications through a service, you must implement it as a foreground service.
For information about implementing notifications with foreground services, see Services that show a notification immediately.
-
Notifications are not shown when the watch is muted or in Do Not Disturb (DND) mode.
-
For watch applications supporting Android 13 and higher:
-
To display notifications, the application must have the
POST_NOTIFICATION
permission. -
The grayscale small icon must be used as the notification icon, as defined by the Android platform.
-
-
To display notifications, your application must be given permission to do so through the Galaxy Wearable application on the user’s phone. To check that notifications are enabled, in the Galaxy Wearable application, go to "Watch Settings > Notifications > App Notifications," and make sure the application is enabled in the list.
Sample Application
To see for yourself how notifications work, download the following sample application and install it on any Galaxy Watch running Wear OS Powered by Samsung.
On the phone connected to your Galaxy Watch, in the Galaxy Wearable application, make sure that notifications are enabled for the sample application.
Wear the watch and run the application. To create a notification, tap the "Create notification" button. A notification icon appears as the notification is generated. You can expand the notification to read its message.
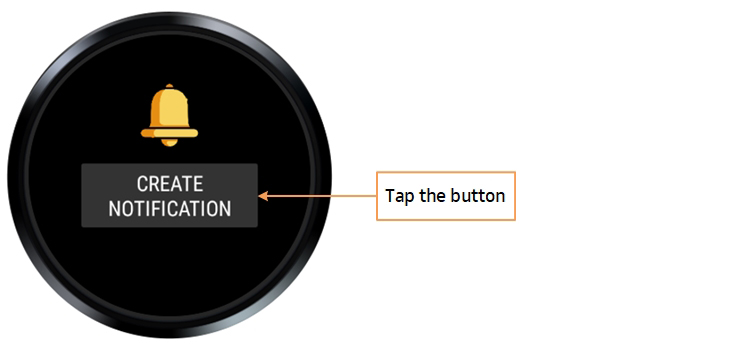
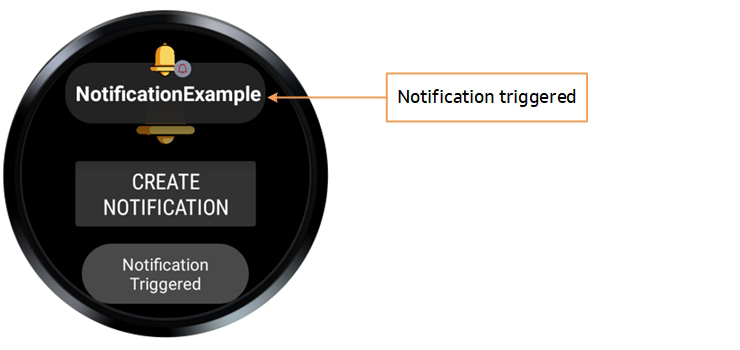
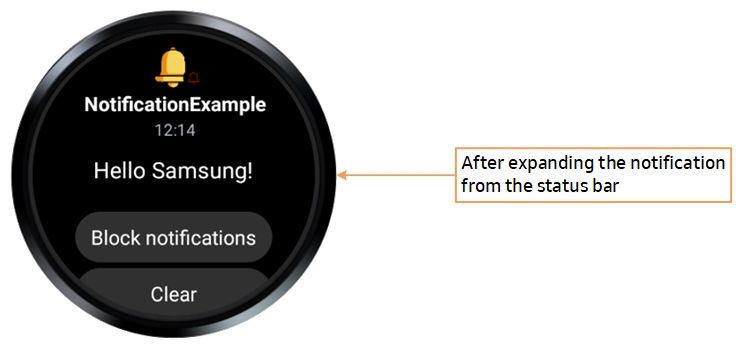
Summary
Implementing notification messages on a Galaxy Watch running Wear OS Powered by Samsung is simple and helps your application inform and engage users. By being aware of the various policies related to watch application notifications, you can ensure that the notifications are displayed successfully.
If a problem persists after following all the above steps, contact Samsung Developer Support for assistance.