Detect When a Galaxy Watch Is Being Worn
Shamima Nasrin
Engineer, Samsung Developer Program
Some features on Galaxy Watch running Wear OS powered by Samsung function only when the watch is being worn on the user’s wrist. Notifications are an important example of one such feature. When the watch is not being worn, phone notifications are not synchronized to the watch and local notifications generated on the watch are muted.
Off-Body detection enables you to determine if the user is wearing the watch. To enable this, the watch contains a Low Latency Off-Body sensor. The data from this sensor allows you to enhance your application by implementing it to, for example, send or avoid notifications or to show or hide sensitive information, as appropriate.
This tutorial demonstrates how to detect if the watch is being worn, and a sample application is provided so you can examine how the code works in practice. The tutorial also describes how you can override the off-body sensor for testing purposes.
Implementing off-body detection for Galaxy Watch
To access the Low Latency Off-Body sensor data on a Galaxy Watch running Wear OS powered by Samsung, the SensorManager library provides the TYPE_LOW_LATENCY_OFFBODY_DETECT
key, which enables you to check if the watch is being worn.
To detect if the watch is being worn:
-
In Android Studio, to create a wearable application project, select "New Project > Wear OS > Blank Activity > Finish."
-
Because the Low Latency Off-body sensor is a body sensor, in the application manifest file, define the required permission to use the body sensors:
<uses-permission android:name="android.permission.BODY_SENSORS" />
-
For Android 6 (API level 23) and higher, to access sensor-related information, the application must obtain permission from the user. In the application code, check that the user has granted access to sensor data:
if (checkSelfPermission(Manifest.permission.BODY_SENSORS) != PackageManager.PERMISSION_GRANTED) { requestPermissions( new String[]{Manifest.permission.BODY_SENSORS}, 1); } else { Log.d(TAG, "ALREADY GRANTED"); }
If the user has not yet granted permission to access sensor data, they are prompted to do so. Your application can extract sensor information only if the user selects "Allow." If they select "Deny," the application cannot access any information from the sensor.
For more information about runtime permissions, see Request permissions.
-
In the application code, create an instance of the
SensorManager
class:mSensorManager = (SensorManager) getSystemService(getApplicationContext().SENSOR_SERVICE);
-
Implement the Low Latency Off-Body sensor:
offBodySensor = mSensorManager.getDefaultSensor(Sensor.TYPE_LOW_LATENCY_OFFBODY_DETECT);
-
To implement an event listener to notify when the sensor value changes, override
onSensorChanged()
:float offbodyDataFloat = sensorEvent.values[0]; int offbodyData = Math.>round(offbodyDataFloat); if (offbodyData == 0) { mTextView.setText("The watch is not being worn!"); mTextView.setTextColor(Color.parseColor("#FF0000")); } else { mTextView.setText("The watch is being worn!"); mTextView.setTextColor(Color.parseColor("#76BA1B")); }
When the
offbodyData
value is 1, the watch is on the user’s wrist. Otherwise, its value is 0, which means the watch is not being worn. -
To activate the listener when the application is running, register it by overriding the
onResume()
method:mSensorManager.registerListener(MainActivity.this, offBodySensor, SensorManager.SENSOR_DELAY_NORMAL);
Alternatively, you can implement registering the listener through a button in the application UI.
-
When the listener is no longer needed, unregister it by overriding the
onPause()
method:mSensorManager.unregisterListener(this);
Alternatively, you can implement unregistering the listener within the
onPause()
method, or through a button in the application UI.
Sample Application
To see for yourself how the off-body sensor works, download the following sample application that supports Galaxy Watch4 or higher.
Extract the application files and open the "OffbodySensorExample/build.gradle" file in Android Studio.
To run the sample application on a Galaxy Watch4 or higher, enable USB debugging mode on the watch. Connect the watch to your computer and run the application through Android Studio.
When you have granted the application permission to access the watch’s sensor data, tap the "Check" button to check if the watch is currently being worn.
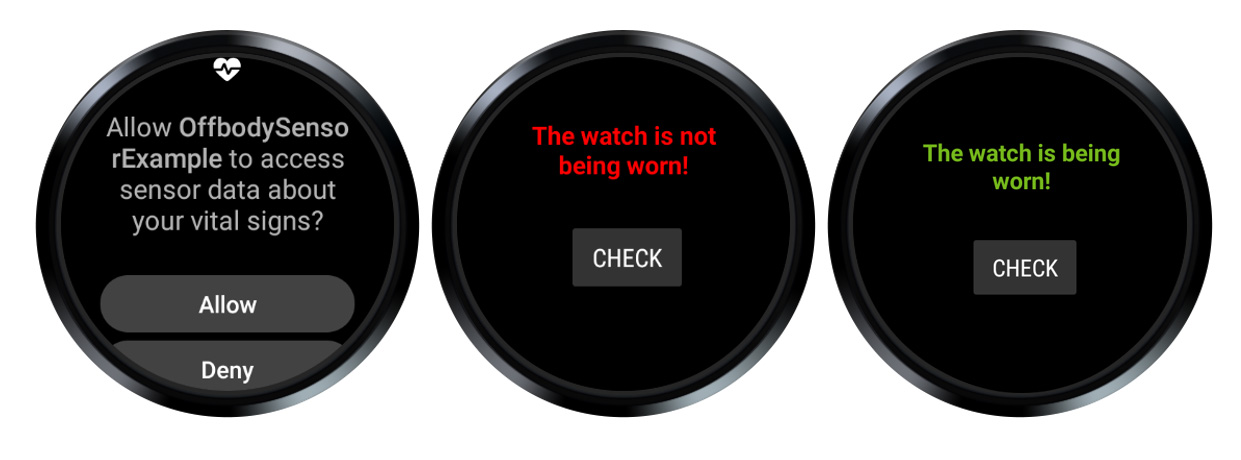
Manually overriding the off-body sensor for testing
During application development, it can be inconvenient to wear the watch while testing. For testing purposes, you can manually override the off-body sensor:
-
Connect the watch to your computer.
-
In the ADB shell, to override the off-body sensor manually:
-
Wearing the watch
$ adb shell am broadcast -a com.samsung.android.hardware.sensormanager.service.OFFBODY_DETECTOR --ei force_set 1
-
Not wearing the watch
$ adb shell am broadcast -a com.samsung.android.hardware.sensormanager.service.OFFBODY_DETECTOR --ei force_set 2
When the command is successful, you see a message similar to "Broadcast completed: result=0."
-
-
To receive notifications, make sure the watch is not in charging mode:
a. To simulate disconnecting the charger, use the following ADB command:
dumpsys battery unplug
b. To re-enable detecting the watch’s charging state normally:
dumpsys battery reset
-
Disconnect the watch from your computer and test your application.
-
After testing, return the watch to its default state. To disable the sensor override, use the following ADB command:
$ adb shell am broadcast -a com.samsung.android.hardware.sensormanager.service.OFFBODY_DETECTOR --ei force_set 0
Summary
Various Galaxy Watch features, such as notifications, work only when the user is wearing the watch. The Low Latency Off-Body sensor enables you to implement application features that react to if the watch is being worn. To ease application development, you can override the sensor so the watch does not need to be physically worn during testing.
If you have questions about or need help with the information in this tutorial, you can contact Samsung Developer Support.