Using a Custom Font In Your Xamarin.Forms Tizen Application
Kangho Hur
Principal Engineer
Previously, in order to use custom fonts in existing Xamarin.Forms applications, you had to add the font asset in each platform project and use the FontFamily
property to apply the fonts. This was very cumbersome, because each platform required a different syntax for referencing a font file.
As we introduced in a previous blog post, using custom fonts in your Tizen .NET applications was not so simple, either.
Xamarin.Forms 4.5.0 introduced a new uniform way to specify custom fonts across platforms. You could define custom fonts on each platform and reuse them by including them as Application Resources
, which was a feature available in Xamarin.Forms 4.5.0 Pre Release 1. We've added Tizen support to this feature, so you don't need to write code to use custom fonts.
NOTE: This change is effective as of Xamarin.Forms 4.5.0 Pre Release 2 and higher.
This blog explains how to use custom embedded fonts in Xamarin.Forms Tizen applications.
Add the font file to your project in Visual Studio
To add fonts as resources, perform the following steps in the Visual Studio.
1. Create a folder to store the font files
To create a folder for storing the font files, right-click the project folder and select Add > New Folder.
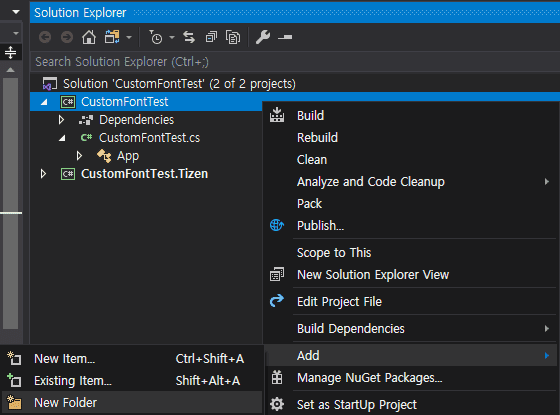
Let's call it Resource.
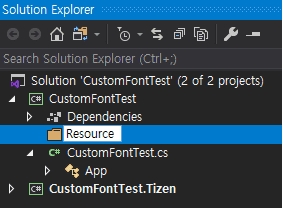
2. Add a font file to your project
To add a font file to your project, right-click the Resource folder you just created and go to Add > Existing item..., or drag the file from File Explore (on Windows) or Finder (on Mac) and drop it into the Resource folder. You can add TrueType font (.ttf
) and OpenType font (.otf
) files. You can obtain sample font files here.
Note: Be sure to add the font file with Build Action: EmbeddedResources
. Otherwise, the font file will not be distributed as part of your app.
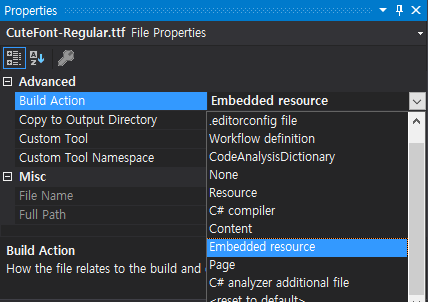
In addition, you should register the font file with the assembly, in a file such as AssemblyInfo.cs
, using the ExportFont
attribute as follows:
using Xamarin.Forms;
[assembly: ExportFont("CuteFont-Regular.ttf")]
[assembly: ExportFont("PTM55FT.ttf")]
[assembly: ExportFont("Dokdo-Regular.ttf")]
[assembly: ExportFont("fa-regular-400.ttf")]
After you add the font file to your project as a Resource
, you can begin assigning the font to VisualElement
items that have the FontFamily
property, such as Label
, Entry
, and so on. Font name can be specified in XAML or in C# code. As mentioned, you no longer need to know platform-specific rules for specifying FontFamily
attributes. It works whether you use the full path and font name separated by a hash (#) as the font name (as in Android) or just the font name (as in iOS).
Note: The font file name and font name may be different. To discover the font name on Windows, right-click the .ttf
file and select Preview. You can then determine the font name from the preview window.
Use your custom font in XAML
<Label Text="Custom Font 한글" />
<!-- Custom Fonts -->
<Label Text="Custom Font 한글" FontFamily ="CuteFont-Regular" />
<Label Text="Custom Font 한글" FontFamily ="Dokdo-Regular" />
<Label Text="Custom Font 한글" FontFamily ="PTM55FT.ttf#PTMono-Regular" />
<Label Text="Custom Font 한글" FontFamily ="fa-regular-400.ttf#FontAwesome5Free-Regular" />
<!-- System Fonts -->
<Label Text="Custom Font 한글" FontFamily ="BreezeSans-Thin" />
<Label Text="Custom Font 한글" FontFamily ="BreezeSans-Bold" />
Use your custom font in C#
new Label
{
Text = "Custom Font 한글",
};
// Custom Fonts
new Label
{
Text = "Custom Font 한글",
FontFamily = "CuteFont-Regular"
};
new Label
{
Text = "Custom Font 한글",
FontFamily = "Dokdo-Regular"
};
new Label
{
Text = "Custom Font 한글",
FontFamily = "PTM55FT.ttf#PTMono-Regular"
};
new Label
{
Text = "Custom Font 한글",
FontFamily = "fa-regular-400.ttf#FontAwesome5Free-Regular"
};
//System Fonts
new Label
{
Text = "Custom Font 한글",
FontFamily = "BreezeSans-Thin"
};
new Label
{
Text = "Custom Font 한글",
FontFamily = "BreezeSans-Bold"
};
All the examples above are also found here.
Screenshot
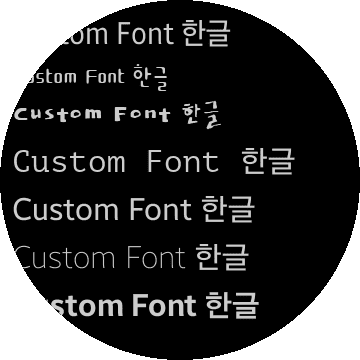
Now that you've reviewed the simple steps in this blog, check out the sample font files, see the many TrueType and OpenType fonts that are out there, and try custom fonts in your own Xamarin.Forms Tizen applications.