Check Which Sensor You Can Use in Galaxy Watch Running Wear OS Powered by Samsung
Shamima Nasrin
Samsung Developer Program Team
Samsung has introduced Galaxy Watch4, a new wearable device that is integrated with Google’s Wear OS, unleashing a new era of wearable technology. The device’s vast sensor lineup provides the user with everyday health related data, resulting in better user experiences and improved capabilities.
Sensors are one of the main attractions for all developers of wearable applications.
There are two special cases that can happen during sensor implementation. Firstly, a device may or may not support a particular sensor. Secondly, some sensors may not be accessible by third-party applications on a particular device.
Therefore, you need to check whether a particular sensor is accessible or not before launching the application. If the application cannot receive data from a particular sensor, you must inform the user. The application layout can also be modified for a better user experience.
The body sensors of wearable devices provide highly advanced data that helps to monitor the condition of the human body. Health applications collect health data from these sensors. The BODY_SENSORS package allows an application to access body sensors, such as heart rate. Although Wear OS Android APIs are available for Galaxy Watch4, you might be unsure if specific body sensor data is accessible for your application on Galaxy Watch.
In this article, I show you how to check if a particular body sensor is accessible on Galaxy Watch4.
Get started
In the following example, I develop a wearable app for Galaxy Watch4 that shows whether a particular sensor is accessible or not.
Environment
Wear OS applications have to be developed using the Android Studio IDE. Here, I am assuming you have already successfully installed Android Studio on your PC.
To start developing a new wearable application, open Android Studio and select New Project > Wear OS > Blank Activity > Finish. The new application project is now ready for you to create your application.
Step-By-Step example of checking sensor accessibility
Step 1: Galaxy Watch4 only supports API level 28 and above. Therefore, you need to set the Target API Level at 28 or higher to develop a wearable application for it. To do this, you can set the Minimum SDK version while creating your project, or you can modify the value in build.gradle > minSDK after the project has been created.
Step 2: The most important part of sensor implementation is to set the appropriate permission. To do this, you have to add the BODY_SENSORS permission in the Android manifest.
<uses-permission android:name="android.permission.BODY_SENSORS" />
Step 3: Starting from Android version 6 (API level 23), an application has to request runtime permission from the user to access any sensor data. If the user grants the permission, the application is then able to access the data.
Add the following code to the onCreate()
method to request user permission:
if (checkSelfPermission(Manifest.permission.BODY_SENSORS) != PackageManager.PERMISSION_GRANTED) {
requestPermissions( new String[]{Manifest.permission.BODY_SENSORS}, 1);
} else {
Log.d(TAG, "ALREADY GRANTED");
}
In the output, a pop-up is shown while installing the application for the first time. If the user selects “Allow,” the application gets permission to access the BODY_SENSORS library information and the pop-up does not reappear when the application is launched again.
If the user selects “Deny,” the application is not able to get the sensor data.
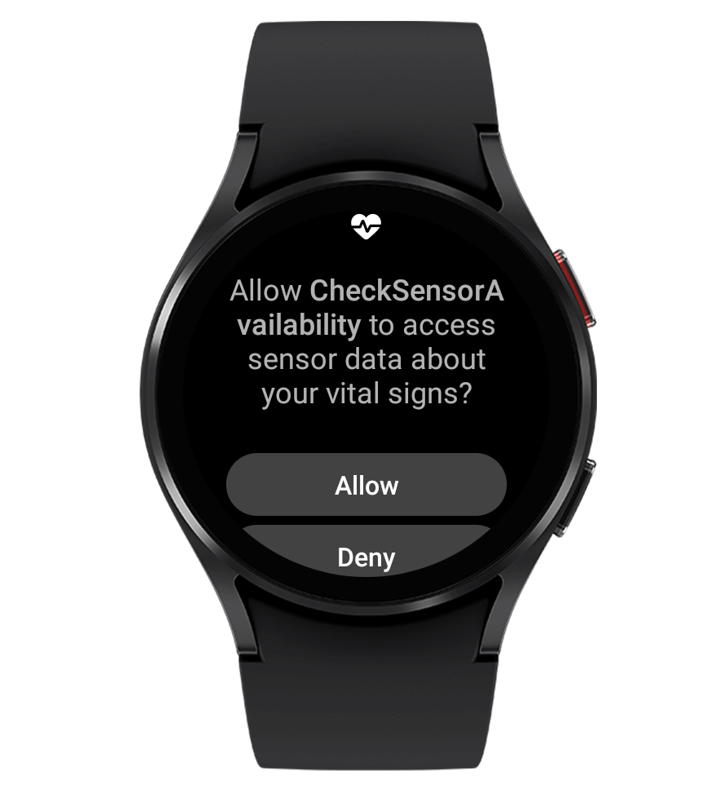
For more details about runtime permissions, check here.
To use the step counter or step detector sensor, you also need the ACTIVITY_RECOGNITION runtime permission. For more information, you can check the following links:
Step 4: Import the following libraries to access sensor data in the Java class:
import android.hardware.Sensor;
import android.hardware.SensorManager;
You can also press Alt + Enter when selecting a particular function to import the required packages automatically.
Step 5: Create an object of the SensorManager class and instantiate it:
SensorManager mSensorManager = ((SensorManager)getSystemService(SENSOR_SERVICE));
Step 6: To check the list of sensors integrated with Galaxy Watch4, add the following code:
List<Sensor> sensors = mSensorManager.getSensorList(Sensor.TYPE_ALL);
ArrayList<String> arrayList = new ArrayList<String>();
for (Sensor sensor : sensors) {
arrayList.add(sensor.getName());
}
arrayList.forEach((n) -> System.out.println(n));
Output of the above code snippet:
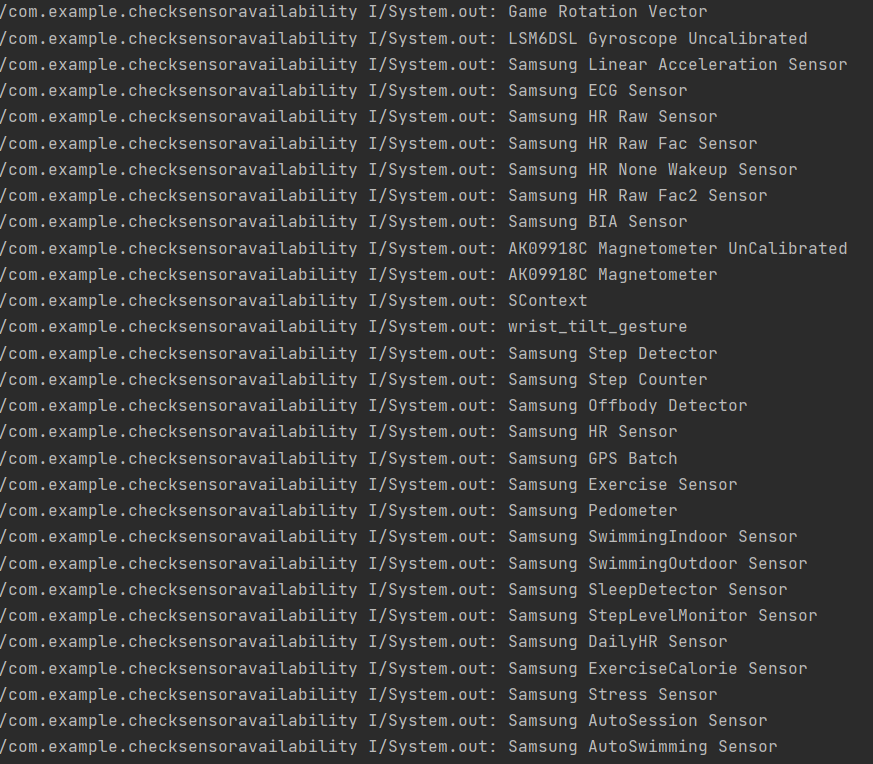
In the output, you can see all the sensors available in Galaxy Watch4 (but remember that sensors which appear in the logcat output may not be accessible for third-party developers) and you can also see the names of private sensors integrated within the watch. Some particular sensors are only accessible from third-party applications.
Note that the only real, hardware sensors in Galaxy Watch4 are the accelerometer, gyroscope, pressure, light, magnetic, and heart rate sensor (PPG). All other sensors are composites of actual hardware sensors. For example, the pedometer sensor combines data from the accelerometer and gyroscope sensors. The legacy SensorManager APIs only work for real physical sensors; composite sensors will not work.
Step 7: Check the accessibility of a particular sensor using the following code:
if ((mSensorManager.getDefaultSensor(Sensor.TYPE_HEART_RATE)) != null) {
// if the sensor is accessible, then do something
} else {
// if the sensor is inaccessible, then do something
}
If the sensor is not accessible on Galaxy Watch4 from a third-party application, the method returns a null value. In this example, we have checked the heart rate sensor using the Sensor.TYPE_HEART_RATE
API. You can check any other sensor by placing your sensor type in this function.
Note that the above process is a way of getting raw sensor data directly from the watch. If you want to get processed sensor data using an API, you can use the Samsung Privileged Health SDK instead.
Moreover, as all sensors are not open for third-party developers and only the actual physical sensors are accessible using SensorManager, it is recommended to use the Samsung Privileged Health SDK. Through this SDK, you can also get some rare sensor data (processed) like SpO2, body composition, ECG, PPG and so on, which is not accessible through SensorManager.
Testing
You can check out the sample app (download it using the link below) and try it out on your Galaxy Watch4.
To import the sample application, open Android Studio and go to File > Open > CheckSensorAvailability > build.gradle.
To run the sample application, connect your Galaxy Watch4 with your PC and run the application. Make sure the USB debugging is set to On in your watch.
In this sample application, we have checked four sensors. Here, the heart rate and pressure sensors (marked in green) are accessible from a third-party application on Galaxy Watch4. The other two sensors, proximity and heartbeat (marked in red), are not accessible.
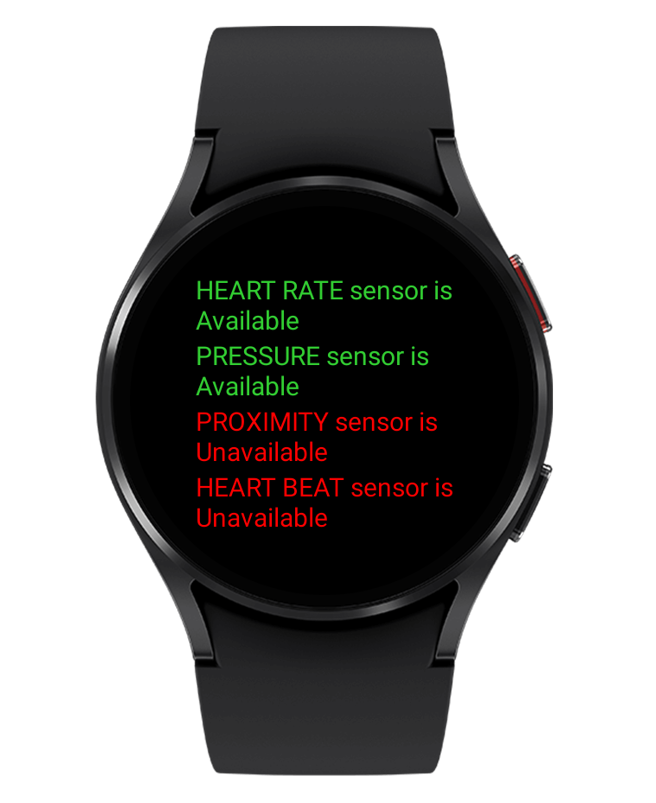
Conclusion
We have demonstrated a way you can check the accessibility of sensors on Galaxy Watch4. If you want to develop any wearable app for Wear OS devices using body sensor data, it is recommended to first check if the data is accessible to third-party applications on the device.