Integrate Samsung IAP in Your Unreal Engine 5 Game
Mobassir Ahsan
Engineer, Samsung Developer Program
Galaxy Store, a default app store bundled with millions of Samsung Galaxy devices, is a top destination for gamers. It has a highly customizable IAP (in-app purchase) system that supports three types of IAP items:
-
Consumables, which are used once and must be repurchased to use again
-
Non-consumables, which has unlimited uses after purchase
-
Subscriptions, which allows unlimited uses for a fixed duration
The Samsung IAP plugin supports Unreal Engine, an advanced 3D game engine with next-generation graphics and features, popular among professional game developers. Unreal Engine 5 is officially supported since Samsung IAP plugin version 6.1.4, enabling you to integrate Samsung IAP into your Unreal Engine games.
This article demonstrates how you can integrate the Samsung IAP plugin into Unreal Engine games to enable users to purchase IAP items.
Prerequisites
The Samsung IAP plugin version 6.1.4 supports Unreal Engine versions 4.18 and higher. Basically, if your tools can build and run an Unreal Engine 5 game for Android devices, you can implement the Samsung IAP plugin features in your game.
The demonstration in this article uses the following recommended development environment:
-
Unreal Engine 5.1
-
Visual Studio 2022
-
Android SDK:
-
Android SDK API Level 32 or higher
-
Android NDK r25b
-
CMake 3.10.2
-
Build tools 33
-
Setting up the development environment
To set up your Unreal Engine game project to implement the Samsung IAP plugin:
- In the Unreal Engine global settings, check that you have defined the target SDK version, minimum SDK version, Android SDK location, Android NDK location, Java location, SDK API level, and NDK API level for your project.
- Open an existing Unreal Engine project or create a new project. The code examples in this article assume an Unreal Engine C++ project.
Go to Edit > Project Settings > Platforms > Android and set the following properties:
- Android Package Name: Define the package name for your project.
- Minimum SDK Version: The version must be 26 or higher.
- Target SDK Version: The version must be 32 or higher.
- Package game data inside apk: Fill the checkbox. This is required because Samsung Galaxy Store Seller Portal only supports uploading single APK files.
Figure 1: Android project settings in Unreal Engine
Download the Unreal IAP plugin from the Samsung Developers website.
Extract the content of the downloaded file to an empty folder inside the Plugins* folder of your project directory. If the <project folder>/Plugins/ folder does not exist, you must create it.
Figure 2: Create the "Plugins” folder
- In the <project folder>/Source/<Project Name>/ folder, open the <project name>.build.cs file.
To add the Samsung IAP plugin to the project dependencies, in the PublicDependencyModuleNames.AddRange() section, add SamsungIAP to the list:
PublicDependencyModuleNames.AddRange(new string[] { "Core", "CoreUObject", "Engine", "InputCore", "EnhancedInput", "SamsungIAP" });
- To enable the plugin within Unreal Engine, relaunch Unreal Engine. Go to Edit > Plugins > Installed > Service and fill the checkbox next to Samsung IAP Plugin.
Relaunch Unreal Engine to ensure the plugin is enabled and ready to use in your project.
Figure 3: Enable Samsung IAP Plugin in Unreal Engine
Registering the game and IAP items
Before Samsung IAP functionalities can be integrated into your game, the game and its IAP items must be registered in Samsung Galaxy Store Seller Portal:
-
In Unreal Engine, go to Edit > Project Settings > Platforms > Android and make sure you have defined the Android Package Name for the project and that Package game data inside .apk is enabled.
-
To build the .APK file, in the Unreal Engine toolbar, select Platforms > Android > Package Project.
-
Register your game application in Samsung Galaxy Store Seller Portal, filling in the required information.
-
In the Binary tab of Seller Portal, upload your game’s APK file.
-
To enable testing the IAP functionalities while developing the game, create a closed beta test. In the Binary tab, select Add Beta Test and register your testers and feedback channel for the closed beta test. For more information, see Beta test.
-
In the In-App Purchase tab, create your IAP items and activate them. For demonstration purposes, the game in this tutorial has two consumable items: "buySuperSpeed" and "buySuperJump."
-
To save your game and IAP item details, select Save.
For more information about registering applications and in-app items, see Register an app and in-app items in Seller Portal.
Integrating in-app purchases
Now that the game application and its IAP items have been registered, you can begin implementing the Samsung IAP features in your code.
Step 1: Include the header file
To access the Samsung IAP functions in your project, you must add the header file to the project code:
-
In Unreal Engine, select Tools > Refresh Visual Studio project.
-
To open and edit the project code in Visual Studio, select Tools > Open Visual Studio.
-
Open the C++ code file where you want to implement the IAP functions.
-
Include the Samsung IAP plugin header file in your code.
#include "IAP.h"
Step 2: Set the IAP operation mode
The Samsung IAP plugin has three operation modes:
-
IAP_MODE_TEST: For development and testing.
-
IAP_MODE_PRODUCTION: For public beta and production releases.
-
IAP_MODE_TEST_FAILURE: For testing failure cases. In this mode, all requests return failure responses.
Set the operation mode using the setOperationMode(<IAP MODE>)
function. Since the game is in development, use "IAP_MODE_TEST." The #if PLATFORM_ANDROID
directive ensures multi-platform compatibility by compiling and executing the code block for the Android platform only, avoiding errors that can be caused when compiling for other platforms.
#if PLATFORM_ANDROID
samsung::IAP::setOperationMode(IAP_MODE_TEST);
#endif
Step 3: Create and set a listener class
The Samsung IAP library has four main API functions:
-
getProductDetails()
retrieves a list of products and their details. -
getOwnedList()
retrieves a list of the user’s owned items. -
startPayment()
initiates a purchase. -
consumePurchasedItems()
consumes a purchased item.
Each of these functions requires a listener or callback function that handles the data returned from the IAP library function:
-
onGetProducts()
is the listener forgetProductDetails()
. -
onGetOwnedProducts()
is the listener forgetOwnedList()
. -
onPayment()
is the listener forstartPayment()
. -
onConsumePurchasedItems()
is the listener forconsumePurchasedItems()
.
Create a skeleton listener class with these callback functions.
In the following examples, a C++ class named SamsungIAPListener
has been created in Unreal Engine, generating the SamsungIAPListener.cpp and SamsungIAPListener.h files in the project source directory.
In the SamsungIAPListener.h file, define the class:
#pragma once
#include "CoreMinimal.h"
#include "IAP.h"
class SamsungIAPListener : public samsung::IAPListener {
public:
void onGetProducts(int result, const FString& msg, const std::vector<samsung::ProductVo>& data);
void onGetOwnedProducts(int result, const FString& msg, const std::vector<samsung::OwnedProductVo>& data);
void onPayment(int result, const FString& msg, const samsung::PurchaseVo& data);
void onConsumePurchasedItems(int result, const FString& msg, const std::vector<samsung::ConsumeVo>& data);
};
In the SamsungIAPListener.cpp file, create skeleton code for the callback functions:
#include "SamsungIAPListener.h"
using namespace std;
using namespace samsung;
#if PLATFORM_ANDROID
void SamsungIAPListener::onGetProducts(int result, const FString& msg, const vector<ProductVo>& data) {
}
void SamsungIAPListener::onGetOwnedProducts(int result, const FString& msg, const vector<OwnedProductVo>& data) {
}
void SamsungIAPListener::onPayment(int result, const FString& msg, const PurchaseVo& data) {
}
void SamsungIAPListener::onConsumePurchasedItems(int result, const FString& msg, const vector<ConsumeVo>& data) {
}
#endif
In the main code file, to set the SamsungIAPListener
listener class that was just created as the IAP listener class for the project, use the setListener()
function.
#include "SamsungIAPListener.h"
#if PLATFORM_ANDROID
samsung::IAP::setOperationMode(IAP_MODE_TEST);
samsung::IAP::setListener(new SamsungIAPListener);
#endif
Step 4: Retrieve purchasable items
To retrieve the list of available IAP items, use the getProductDetails()
function:
samsung::IAP::getProductDetails("");
The IAP library returns the available items list in the parameters of the onGetProducts()
callback function. The data variable contains the list, from which you can extract its member variables and handle them in Unreal Engine as needed.
For instance, you can use the onGetProducts()
callback function to display the list of available items and their prices in the game:
void SamsungIAPListener::onGetProducts(int result, const FString& msg, const vector<ProductVo>& data) {
for (auto& i : data) {
printMessage(FString::Printf(TEXT("%s Price = %s"), *i.mItemName, *i.mItemPriceString));
}
}

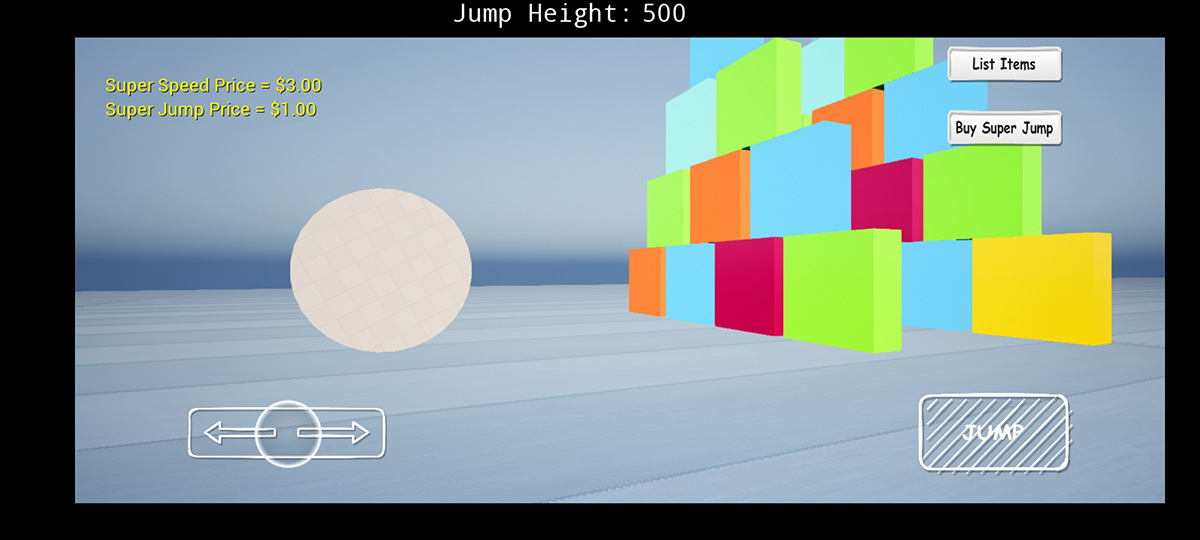



Figure 4: List of available items retrieved

You can also retrieve the details for a specific item by placing its item ID in the string parameter of the getProductDetails()
function. For example, getProductDetails("buySuperJump")
retrieves only the details for the buySuperJump item.
Step 5: Retrieve owned items
To ensure that the user’s previously purchased IAP items are available in the game, when the game is launched, retrieve the list of items that they own, using the getOwnedList()
function:
samsung::IAP::getOwnedList(PRODUCT_TYPE_ALL);
The onGetOwnedProducts()
callback function receives the owned item list. You can use it to perform tasks such as restoring the purchased items’ functionalities in the game, and checking for and consuming any consumable items in the user’s account that have not been consumed yet. It is important to consume the consumable items as soon as possible, because they cannot be repurchased until they are consumed.
void SamsungIAPListener::onGetOwnedProducts(int result, const FString& msg, const vector<OwnedProductVo>& data) {
if (data.size() == 0) {
printMessage(FString::Printf(TEXT("No previously owned items")));
}
else {
for (auto& i : data) {
samsung::IAP::consumePurchasedItems(*i.mPurchaseId);
printMessage(FString::Printf(TEXT("Consuming Owned Item: %s"), *i.mItemName));
}
}
}
Step 6: Purchase items
Initiate purchasing an item with the startPayment()
function. In this example, the user wants to purchase the item with the item ID “buySuperJump:”
samsung::IAP::startPayment("buySuperJump", "pass_through_value", true);
When the function is called, the screen switches to portrait mode and displays the checkout UI. The user selects their payment method and confirms the payment. When Galaxy Store successfully processes the payment, the item is added to the user’s owned item list. To check whether the item has been added to the user’s owned item list, you can use the getOwnedList()
function.

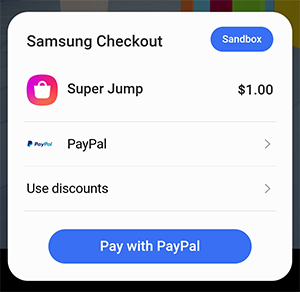



Figure 5: Samsung IAP Checkout UI

Remember that consumable items remain on the user’s owned item list and cannot be repurchased until the item is consumed. Ideally, apply the item’s game effect and consume the item as soon as it is purchased. Since the result of the startPayment()
function is returned to the onPayment()
callback function, you can extend this callback function to do tasks with the item after completing the payment.
In our example game, the variable jumpHeight is set to 500. When the user buys the “buySuperJump” item, the jump height is doubled. This game effect can be implemented within the onPayment()
callback function.
void SamsungIAPListener::onPayment(int result, const FString& msg, const PurchaseVo& data) {
FString sJump = TEXT("buySuperJump");
if(sJump.Equals(*data.mItemId)){
jumpHeight = 1000;
superJumpsAvailable++;
samsung::IAP::consumePurchasedItems(*data.mPurchaseId);
printMessage(FString::Printf(TEXT("Super Jump Purchased")));
}
}
Step 7: Consume purchased items
To consume a previously purchased item, you must first retrieve its purchase ID. The purchase ID can be found within the data variable in the callback function for the startPayment()
and getOwnedList()
functions. You can implement consuming the item directly from the callback function with the consumePurchasedItems()
function, in the same way as previously demonstrated.
samsung::IAP::consumePurchasedItems(*data.mPurchaseId);
When an item is consumed through the consumePurchasedItems()
function, the onConsumePurchasedItems()
callback function is triggered. In this callback function, make sure that the result of the purchase has been stored locally before the IAP item is consumed and removed from the user’s owned item list.
void SamsungIAPListener::onConsumePurchasedItems(int result, const FString& msg, const vector<ConsumeVo>& data) {
for (auto& i : data) {
printMessage(FString::Printf(TEXT("Item Successfully Consumed")));
}
}
Results and summary
With the Samsung IAP feature fully integrated into our example Unreal Engine game, we can test the IAP functionality within the game.
Purchasing the “buySuperJump” item triggers a chain of IAP library functions that acquires the item and consumes the item to implement its game effect:
-
startPayment()
function initiates the payment process. -
onPayment()
callback function reacts to the successful payment:a. Changes the user’s jump height in the game to 1000.
b. Displays the message “Super Jump Purchased.”
c. Calls the
consumePurchasedItems()
function. -
consumePurchasedItems()
function consumes the purchased item, removing it from the user’s owned items. -
onConsumePurchasedItems()
callback function reacts to consuming the item, displaying the message “Item Successfully Consumed.”

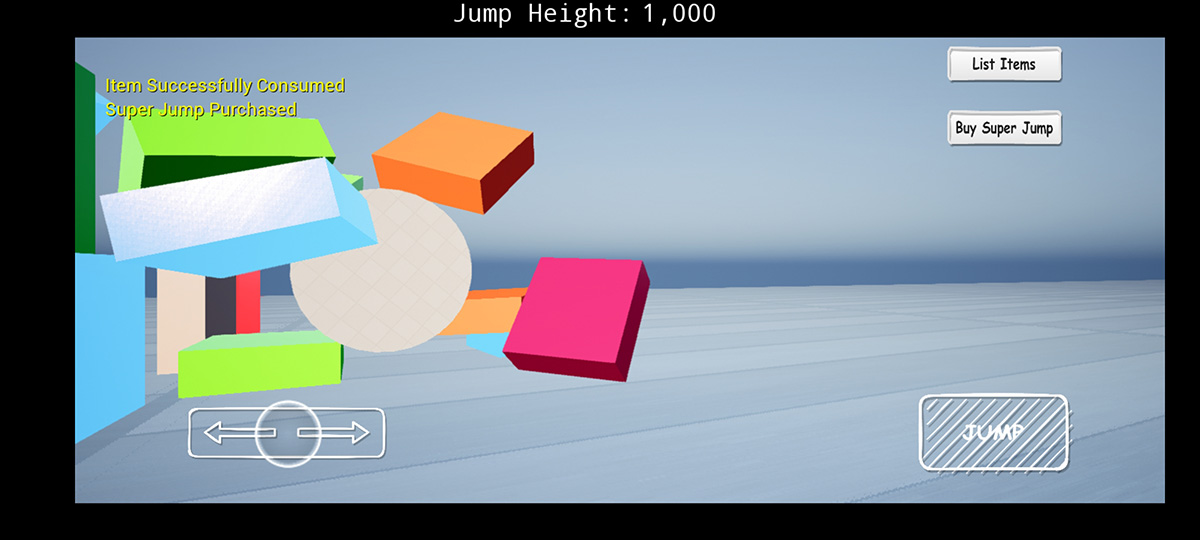



Figure 6: “buySuperJump” item successfully purchased and consumed

This demonstration has shown how easy it is to integrate Samsung IAP in an Unreal Engine 5 game. With only a few library functions, you can implement any Samsung IAP functionalities that you want in games made with Unreal Engine.
To learn more about how Samsung IAP works, see our previous article Integration of Samsung IAP Services in Android Apps. If you have questions about or need help with the information in this article, you can share your queries on the Samsung Developers Forum.