Payment Card Push Provisioning Using Samsung Pay SDK
Yasin Hosain
Engineer, Samsung Developer Program
Samsung has invested tremendous effort in the digital economy from the very beginning and continuously facilitates millions of people who use Samsung Wallet. Samsung Wallet is one of the most used digital wallets right now in the cashless industry. It is a medium that fuels up digital currency transactions by its ease-of-use and security.
To support various businesses, Samsung has developed the Samsung Pay SDK which allows you to use Samsung Wallet’s features effortlessly. The Samsung Pay Android SDK consists of Android-based APIs to perform transactions using Samsung Wallet. It helps to implement simple payment solutions, which boost your businesses' growth to the next level. To understand the business and possibilities, visit the Samsung Pay SDK portal for more information.
What is push provisioning?
Push provisioning is one of the major features of the Samsung Pay SDK. It allows an issuer bank to push the payment experience to a digital wallet. It simplifies the process of adding a payment card as it can be done with a single tap of a finger.
The normal process is time consuming since it involves multiple steps, and the user needs to enter their card information. With push provisioning, the process is easier as it can be done with a single tap, and it's also secure because the process is performed from the issuer application.
This tutorial demonstrates how to implement the push provisioning feature in an issuer application with the Samsung Pay SDK.

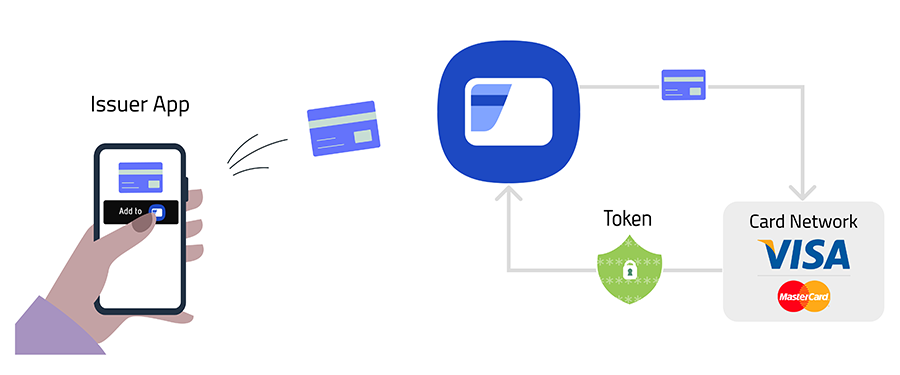



Figure 1: Samsung Pay push provisioning concept

Prerequisite
To use the Samsung Pay SDK, you must be an official Samsung partner. For information about the partnership process, see the Samsung Pay member guide. As a Samsung Pay SDK partner, you can utilize this tutorial while implementing push provisioning.
SDK integration
-
To download the Samsung Pay SDK, go to Samsung Developers Portal > Samsung Pay > Download . When the download is complete, a .jar file called “SamsungPaySDK_x.xx.xx_release.jar” can be found under the Libs folder.
-
Add the Samsung Pay SDK .jar file to the libs folder of your Android project using Android Studio or File Explorer.
-
Navigate to the build.gradle file of your application module and enter the following dependency to the dependencies block:
implementation files('libs/SamsungPaySDK_x.xx.xx_release.jar')
-
If the target SDK version is 30 or higher (Android 11 or R-OS), include the following <queries> element in AndroidManifest.xml. For more information, see Declare package visibility needs.
<queries> <package android:name="com.samsung.android.spay" /> </queries>
-
Since we are using the latest SDK, set the API level to 2.18. We recommend using the updated version of the Samsung Pay SDK for a better user experience. Implement the following code in the <application> element of AndroidManifest.xml:
<meta-data android:name="spay_sdk_api_level" android:value="2.18" /><!-- most recent SDK version is recommended to leverage the latest APIs-->
-
Configure the debug or release mode for your project as follows:
-
For testing and QA, set the debug mode value to Y. This mode requires the allowed Samsung account(s) list to test.
-
For market release to end users, set the debug mode value to N.
<meta-data android:name="debug_mode" android:value="N" /><!--set to Y if debug mode-->
-
Let’s get started
Since the main functionalities in this tutorial are based on push provisioning, we need to initialize the SamsungPay and CardManager classes first. These two classes take the same parameters to construct, which are Context and an instance of the PartnerInfo class. The PartnerInfo object contains information about partners, which is to validate the request from the issuer application.
private val partnerInfo = PartnerInfoHolder.getInstance(context).partnerInfo
private val samsungPay= SamsungPay(context,partnerInfo)
private val cardManager = CardManager(context, partnerInfo)
Check if Samsung Wallet is ready
Since the Samsung Pay SDK depends on Samsung Wallet, you need to ensure that Samsung Wallet is prepared for transactions. Check the Samsung Wallet status before the SDK API call using the getSamsungPayStatus()
method. If you get the "SPAY_READY" status, you can proceed further to call any API. For more details, see the programming guide.
samsungPay.getSamsungPayStatus(object : StatusListener {
override fun onSuccess(status: Int, bundle: Bundle?) {
if(status == SpaySdk.SPAY_READY){
//Enable Samsung Pay SDK functionalities
}
else{
//Disable Samsung Pay SDK functionalities
}
}
override fun onFail(status: Int, bundle: Bundle?) {
//Disable Samsung Pay SDK functionalities
}
})
Check if the card is added
During development, you may need to know whether the card is already added to Samsung Wallet or not. To get a list of cards that have already been added to the wallet, use the getAllCards()
method. For card-related operations, such as getting cards from Samsung Wallet, the CardManager class is the responsible one to perform. This means that the getAllCards()
method is a property of the CardManager class. This method takes a Bundle and an interface as parameters, so it returns the result based on the operation.
getSamsungPayStatus()
method must be called before the getAllCards()
method. The getAllCards()
method cannot return a card list if the getSamsungPayStatus()
method responds with a code other than SPAY_READY.getAllCards()
method returns empty data even though a card has been added. The main reason for this issue is that the card issuer name and issuer name on the Samsung Pay portal are not the same. For more information, see the FAQ & Troubleshooting page.val getCardListener: GetCardListener = object : GetCardListener {
override fun onSuccess(cards: List<Card>) {
//Show cards
}
override fun onFail(errorCode: Int, errorData: Bundle) {
//Show error
}
}
cardManager.getAllCards(null, getCardListener)
Configure your payload
The getWalletInfo()
method is designed to request wallet information from the Samsung Wallet application prior to performing the add card operation. Wallet information can be mandatory or optional for token providers for payload configuration. For Visa, wallet information is mandatory, and you have to use it while building the payload.
val keys = ArrayList<String>()
keys.add(SamsungPay.WALLET_DM_ID)
keys.add(SamsungPay.DEVICE_ID)
keys.add(SamsungPay.WALLET_USER_ID)
val statusListener: StatusListener = object : StatusListener {
override fun onSuccess(status: Int, walletData: Bundle) {
val deviceId: String? = walletData.getString(SamsungPay.DEVICE_ID)
val walletAccountId: String? = walletData.getString(SamsungPay.WALLET_USER_ID)
//Build payload
}
override fun onFail(errorCode: Int, errorData: Bundle) {
//Show error
}
}
samsungPay.getWalletInfo(keys, statusListener)
Complete the push provisioning
The last command to execute the push provisioning operation in our tutorial is the addCard()
method, which is required to perform the push provisioning in Samsung Wallet. The payload we have created must be delivered with the addCard()
method as a parameter. The payload is a tricky part where partners can sometimes get confused or misguided. To be clear, Samsung does not provide the payload for push provisioning. The payload is defined between the issuer and the token provider, and it varies based on different issuers and token providers. Samsung only delivers the payload to the token provider without consuming anything. The addCard()
method also takes a callback as a parameter to return the result based on the operation.
val tokenizationProvider = AddCardInfo.PROVIDER_ABCD
val cardDetail = Bundle()
cardDetail.putString(EXTRA_PROVISION_PAYLOAD, payload)
var cardType = Card.CARD_TYPE_CREDIT_DEBIT
val addCardInfo = AddCardInfo(cardType, tokenizationProvider, cardDetail)
val addCardListener: AddCardListener = object : AddCardListener {
override fun onSuccess(status: Int, card: Card) {
//Show successful message
}
override fun onFail(errorCode: Int, errorData: Bundle) {
//Show error
}
override fun onProgress(currentCount: Int, totalCount: Int, bundleData: Bundle) {
//extra event for operation count
}
}
cardManager.addCard(addCardInfo, addCardListener)
Summary
Push provisioning is the powerful experience of sharing information to a digital wallet, and digital wallets transform this experience to the next level by facilitating transactions within seconds. Samsung Wallet handles these operations and helps the digital payment system to its next step.
For more information about the Samsung Pay SDK, visit the Samsung Pay Developers Portal. If you face any issues during SDK implementation, contact the Developer Support team.