App Management Using the Galaxy Store Developer API and Python
Jakia Sultana
Engineer, Samsung Developer Program
If you want to sell your app in Galaxy Store, you need to use Galaxy Store Seller Portal which is the management portal for uploading your app for distribution. However, if you want to perform application management tasks programmatically, then the Galaxy Store Developer (GSD) API is for you. The GSD API is a set of REST APIs that allows you to use the key functions of Seller Portal without having to access the Seller Portal UI.
There are many tasks in the app distribution system which are performed frequently. Managing an app or apps manually for each app store can be a waste of resources for tasks such as releasing an update of a published app. The Content Publish API is a part of the GSD API which allows you to view, modify, submit, and change the status of apps registered in Seller Portal. This API provides programmatic access to the same types of functionality provided by Seller Portal.
Today, I show you how you can release an update of your app by using the Content Publish API. I am going to demonstrate how to implement the Content Publish APIs using Python. However, I also recommend you use CURL to test the APIs which are documented in the Content Publish API guide. Now, let's get started!
Prerequisites:
-
Python: Install Python on your PC.
-
Seller account: Create a seller account in Seller Portal following the instructions in the Galaxy Store Seller Portal User Guide.
-
Service account ID: Get a service account ID from Seller Portal. You can find the details here.
-
Authorization: Get an access token from the Galaxy Store authentication server using the
accessToken
API. Please check out this blog to learn more about creating an access token using Python.
As an example, I have an app called "Flying Birds Attack" which is live in Galaxy Store. Now I want to publish an update to this app that fixes some bugs. So, I need to first build the release version of this app, called "app-release.apk," and then I call the following APIs to upload this version for review in Galaxy Store:
-
View seller's app details: I use this API to learn detailed information about the app so that I can use this information while modifying it.
-
Create session ID for file upload: A session ID is required to upload any type of file. The session ID is unique and valid for 24 hours.
-
File upload: Next, I upload the new .apk file by using this API.
-
Modify app data: This API is required to modify the app's information and to add the new binary file.
-
Submit app: Finally, I submit the app for review.
Let's start by writing the Python script. To make an HTTP request to a specified URL, I need the requests
library. So, first I have to import the library in the script. At this point I also import the json
library to see the response in the JSON format.
# Importing the requests library
import requests
# Importing the json library
import json
The following headers are required to call any API:
Attribute | Type | Description |
---|---|---|
Authorization | string | Bearer <your-access-token> |
service-account-id | header | Your service account ID |
So, I set these required header values in the Python script.
access_token = "<access_token>"
Authorization = "Bearer " + access_token
SERVICE_ACCOUNT_ID = "<your-service-account-id>"
The previously mentioned APIs are described below.
View seller's app details
This API returns information about one of the seller's registered apps. The content ID of the target app is required for this API. So, I set the content ID and request URL:
Content_ID = '<Content ID of your app>'
# Defining the api-endpoint
api_url = 'https://devapi.samsungapps.com/seller/contentInfo?contentId=' + Content_ID
Next, let's create a dictionary for the header, as I have to send the previously mentioned data in the header.
# Header to be sent to the API
headers= {
'Authorization': Authorization,
'service-account-id': SERVICE_ACCOUNT_ID
}
payload={}
Finally, I send the HTTP GET request to get detailed information about the target app.
try:
response = requests.get(api_url, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
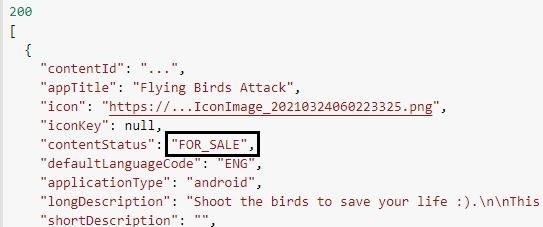
Note that the content status is "FOR_SALE" which will change after registering the binary file.
Create a session ID for file upload
For uploading a file, a session ID is required. Therefore, I call this API to generate a session ID so that I can upload the new binary file. I send the HTTP POST request to create the session ID. In the response object, I only need the "sessionId" value, so this value is stored as "session_ID." The full script is given below:
# Importing the requests library
import requests
access_token = "<access_token>"
Authorization = "Bearer " + access_token
SERVICE_ACCOUNT_ID = "<your-service-account-id>"
api_url = "https://devapi.samsungapps.com/seller/createUploadSessionId"
# Header to be sent to the API
headers= {
'Authorization': Authorization,
'service-account-id': SERVICE_ACCOUNT_ID
}
payload={}
try:
response = requests.post(api_url, headers=headers,data=payload)
print(response.status_code)
data_shows = response.json()
session_ID = data_shows["sessionId"]
print(session_ID)
except Exception as e:
print(str(e))
File upload
I can upload any type of file required for an app submission or for updating an app: a binary file, image (icon, cover image, or screenshot), or zip file. In this example, I send a binary file for updating the app. This API returns a filekey, which is required for updating the app. Here, the session ID is sent as data object and the file is sent as file object.
api_url = "https://seller.samsungapps.com/galaxyapi/fileUpload"
file_path = "<file-path>"
# Header to be sent to the API
headers= {
'Authorization': Authorization,
'Accept': 'application/json',
'service-account-id': SERVICE_ACCOUNT_ID
}
files = {'file': open(file_path,'rb')}
payload = {
'sessionId': Session_ID,
}
try:
response = requests.post(api_url, headers=headers,files=files,data=payload)
print(response.status_code)
print(response.text)
except Exception as e:
print(str(e))
Modify app data
This API updates the content of an app. This API only works after an app has been submitted and is FOR SALE in Galaxy Store. If the app is in the process of verification or if it is in the rejected status, it cannot be updated through this API. The following parameters are required for registering a binary: contentId, defaultLanguageCode, paid, usExportLaws, and binaryList.
The Python script is similar to the view seller's app details
API example above. I just need to change the data to be sent to the API. First, I create a dictionary to hold the data which is sent to the API. In this example, I keep the existing binary and register a new binary. In the JSON structure, I add two sets of binaryList parameters: the first one for the existing binary and the second for adding a new binary. Note that if you want to delete the existing binary, you have to remove the first binaryList parameter.
Note that I need to send serialized data in the form of a string and thus json.dumps
is necessary to perform the serialization.
packageName = "<package-name-of-your-app>"
fileKey = "<new-binary-file-key>"
payload = json.dumps({
"contentId": Content_ID,
"defaultLanguageCode": "ENG",
"paid": "N",
"usExportLaws": True,
"ageLimit": "0",
"chinaAgeLimit":"0",
"binaryList": [
{
"fileName": "MyApp.apk",
"binarySeq": "4",
"versionCode": "5.0",
"versionName": "1.1",
"packageName": packageName,
"nativePlatforms": None,
"apiminSdkVersion": "18",
"apimaxSdkVersion": None,
"iapSdk": "Y",
"gms": "N",
"filekey": None
},
{
"gms": "N",
"filekey": fileKey
}
]
})
Finally, I define the URL and send the POST request.
api_url = "https://devapi.samsungapps.com/seller/contentUpdate"
try:
response = requests.post(api_url, headers=headers,data=payload)
print(response.status_code)
print(response.text)
except Exception as e:
print(str(e))
I can modify the new feature parameters, upload new screenshots, or modify any other parameter as well. You can find details about each kind of modification in the Content Publish API Reference guide.
After registering the binary, I can call the view seller's app details
API to verify the update. In Figure 1, I see that the content status is "FOR_SALE." In Figure 2 below, I can see it has changed to "REGISTERING."
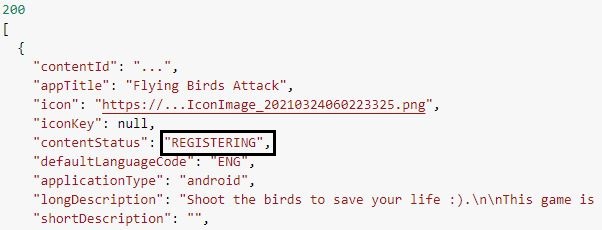
I can also view the changes in Seller Portal. Before calling the content update API, the app is in the "For Sale" state under the Sale Status tab. After calling the content update API, the app is in the "Updating" state under the Registration tab.
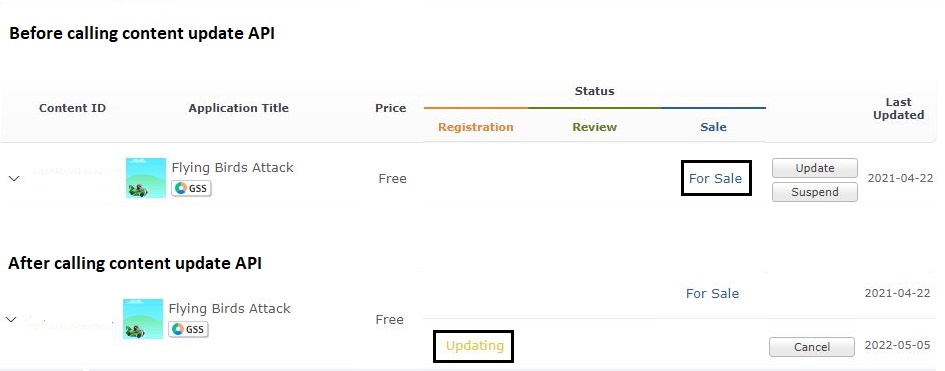
Submit app
This API is for submitting the app for review. An app’s contentStatus attribute must be "REGISTERING" before it can be submitted. In Figure 2, I can see the contentStatus attribute is "REGISTERING" after the new binary was registered, so I can submit the app by using this API.
I set the content ID as data while sending the POST request.
# Defining the api-endpoint
api_url = "https://devapi.samsungapps.com/seller/contentSubmit"
payload = json.dumps({
"contentId": Content_ID
})
try:
response = requests.post(api_url, headers=headers,data=payload)
print(response.status_code)
print(response.text)
except Exception as e:
print(str(e))
The app is successfully submitted. Note that if any parameter remains blank, then this API does not work and you get a check validation (Essential Input) error. To check the current status of the app, I once again call the view seller's app details
API.
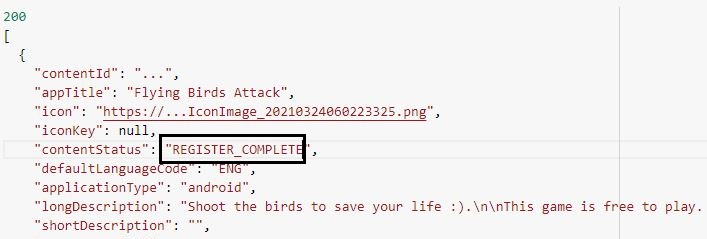
Conclusion
Today, you have learned how to update an app using the Content Publish API. You can use this API in your continuous integration and development system to save time and reduce manual work. I hope you find this article helpful!
Blog articles in this series
-
How to Create an Access Token for the Galaxy Store Developer API Using Python: An access token is sent in the authorization header of every Galaxy Store Developer API call. Learn how to create an access token using the Galaxy Store Developer API and Python.
-
Want Statistics for Your Apps Using the Galaxy Store Developer API?: How to implement the Galaxy Store Statistics APIs using Python to get statistical data about your apps.
-
Managing In-App Items Using the Galaxy Store API and Python: View, create, modify, and remove in-app items using the IAP Publish API and Python.