Managing In-App Items Using the Galaxy Store Developer API and Python
Jakia Sultana
Engineer, Samsung Developer Program
Galaxy Store provides a platform where developers can sell their apps to Galaxy device users. Samsung In-App Purchase (IAP) is a payment service which allows you to sell your app's in-app items that are distributed through Galaxy Store. If you want to make any changes to your app's in-app items, then you have to update your app using Galaxy Store Seller Portal. However, if you want the changes to be reflected immediately within the content, even when the content is in the For Sale state, then the Galaxy Store Developer API is the solution for you.
The Galaxy Store Developer API provides programmatic access to the same types of functionality provided by the Galaxy Store Seller Portal. The IAP Publish API is a part of the Galaxy Store Developer API, a set of server-to-server APIs that allows you to manage in-app items. This API is used to view, register, modify, and remove Samsung IAP items. You can find the CURL commands for this API in the IAP Publish API guide. In this blog article, I am going to demonstrate how to implement the IAP Publish API using Python. Let's get started!
Get Started
In this example, you should already know about the In-App Purchase SDK and how to create items in Seller Portal. To learn how to sell in-app items through Galaxy Store, please read the In-App Purchase programming guide.
The IAP Publish API supports CRUD operations for in-app items: CREATE, READ, UPDATE, and DELETE. The available HTTP methods for these operations are POST, GET, PUT, PATCH, and DELETE, respectively. Later, I explain which HTTP method is used for which IAP Publish API.
Since I implement this API in Python, I need to install Python on my PC. The rest of this blog assumes you have already done this.
Prerequisites:
-
Seller account: Create a seller account in Galaxy Store Seller Portal using the Galaxy Store Seller Portal User Guide.
-
Service account ID: Get the service account ID from Seller Portal. You can find the details here.
-
Authorization: Get the access token from the Galaxy Store authentication server using the
accessToken
API. Check out this blog to learn about creating an access token using Python. -
IAP SDK integrated app: The IAP SDK must be integrated into the app. All binaries registered in the content must support IAP.
-
Connection with the content of the In-App Purchase tab area of Seller Portal: To create this connection, you must either initially distribute your content using the Content Publish API or the content must be in the For Sale state in Seller Portal.
The following headers are required to call any API, just like any other Galaxy Store Developer API.
Attribute | Type | Description |
---|---|---|
content-type | string | application/json |
Authorization | string | Bearer <your-access-token> |
service-account-id | header | Your service account ID |
The IAP Publish API provides a total of six APIs to manage in-app items. After fulfilling the above requirements, I am ready to implement these APIs in Python.
View item list
I can view the list of item information within a scope. While sending this request, I have to specify the number of pages of content to return and the number of items to be returned for a page.
Since this is a REST API, I have to make an HTTP request. To make an HTTP request to a specified URL, I need the requests
library. So, first I have to import the library in our script. I also import the json
library to see the response in JSON format.
# Importing the requests library
import requests
# Importing the json library
import json
As mentioned earlier, I need to send some data as the header in the request. First I set these values before creating the header. Then I create a dictionary using the syntax {key: value}. Here, the key is the attribute name and the value is the header content.
access_token = "<access_token>"
Authorization = "Bearer " + access_token
SERVICE_ACCOUNT_ID = "<your-service-account-id>"
# Header to be sent to the API
headers= {
'Authorization': Authorization,
'content-type': 'application/json',
'service-account-id': SERVICE_ACCOUNT_ID
}
The GET method is used to read or retrieve data from the given server using a given URL. It doesn't change any data. Therefore, I use an HTTP GET request for this API. In the GET request, the package name of an app, the number of pages, and the number of items are required. As an example, I set the number of pages as 1 and the number of items as 20.
packageName = "<package-name-of-your-app>"
page = '1'
size = '20'
Now, I define the URL and send the GET request.
# Defining the API endpoint
item_list = 'https://devapi.samsungapps.com/iap/v6/applications/' + packageName + '/items?page=' + page + '&size=' + size
payload={}
try:
response = requests.get(item_list, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
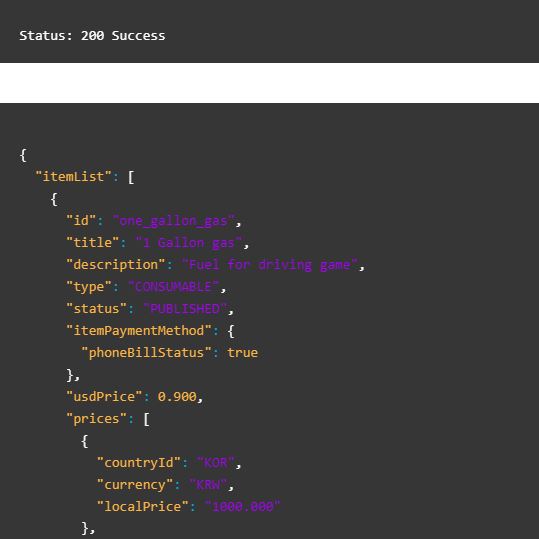
View individual item
I use this API to view the detailed information of one item. The item ID is a required parameter for this API, being a unique identifier of an in-app item registered in Seller Portal. The Python script for this API is similar to the view item list
API; I just need to change the request URL.
itemName = '<item-id-of-specific-package-id>'
# Defining the API endpoint
individual_item = 'https://devapi.samsungapps.com/iap/v6/applications/' + packageName + '/items/' + itemName
try:
response = requests.get(individual_item, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
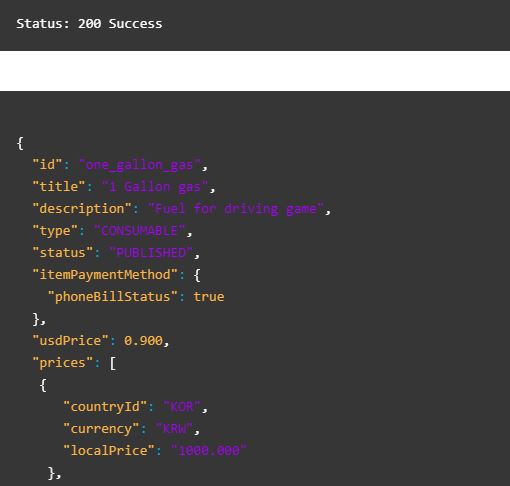
Create item
This API allows you to create a new item, which can be either consumable or non-consumable. To learn more about the item types, visit the IAP Programming guide.
To register an item, you are required to set nine parameters as the data in an HTTP POST request. The POST method is used for creating a new resource.
First, let's go through an example where I create a new item called 'one_gallon_gas'. I set a title of this item and a description which explains the purpose of this item. To be able to purchase the item multiple times, I set its type as consumable. I set the 'phoneBillStatus' attribute as 'true' so that the user can pay by an automatic payment on their phone bill. I set the item status as 'PUBLISHED' to list it for sale in Galaxy Store. Finally, I set the USD price, local price, country ID, and currency values.
Next, I create a dictionary to hold the data which is sent to the API. I need to send serialized data in a form of a string and thus json.dumps
is necessary to perform the serialization.
# Data to be sent
payload = json.dumps({
"id": "one_gallon_gas",
"description": "Fuel for driving game",
"status": "PUBLISHED",
"type": "CONSUMABLE",
"usdPrice": 0.99,
"title": " 1 Gallon Gas",
"prices": [
{
"countryId": "NGA",
"currency": "NGN",
"localPrice": "400.000"
},
{
"countryId": "PER",
"currency": "PEN",
"localPrice": "3.900"
},
{
"countryId": "SWE",
"currency": "SEK",
"localPrice": "11.000"
}
]
})
Finally, I define the URL and send the POST request.
new_item = "https://devapi.samsungapps.com/iap/v6/applications/" + packageName + "/items"
try:
response = requests.post(new_item, headers=headers,data=payload)
print(response.status_code)
#print(response.text)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
Modify item
This API allows you to modify an in-app item without updating the app. The item ID can't be changed using this API. It is recommended to use the response from the view individual item
API to create the input required for this request.
In this example, I modify the title and description of an item which I created in the previous section. I also modify the USD price, local price, country ID, and currency values.
I create a dictionary to hold the data which is sent to the API, as was done in the previous section.
# Data to be sent
payload = json.dumps({
"id": "one_gallon_gas",
"title": "3 Gallon Gas",
"description": "Modify fuel for driving game fix",
"type": "CONSUMABLE",
"status": "PUBLISHED",
"itemPaymentMethod": {
"phoneBillStatus": True
},
"usdPrice": 1,
"prices": [
{
"countryId": "KOR",
"currency": "KRW",
"localPrice": "900"
},
{
"countryId": "USA",
"currency": "USD",
"localPrice": "1"
}
]
})
Since this request is for modification, I send it using a PUT request, which is a method for updating the entire resource. So, I define the URL and send the HTTP request.
# Defining the API endpoint
modify_item = "https://devapi.samsungapps.com/iap/v6/applications/" + packageName + "/items"
try:
response = requests.put(modify_item, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
Partial item modification
This API is used to modify the title, countryId, or localPrice of an in-app item. I can't change the item ID using this API. This API only does a partial update unlike the modify item
API, so an HTTP PATCH request is used. The PATCH request requires only those fields which are to be modified. It doesn't change the other fields of the resource, in contrast to the PUT request which changes the entire resource.
In this example, I modify only the title of the 'one_gallon_gas' item. The Python script remains the same as in the previous section.
# Data to be sent to the API
payload = json.dumps({
"id": "one_gallon_gas ",
"title": "5 Gallon Gas"
})
# Defining the API endpoint
partial_modify_item = "https://devapi.samsungapps.com/iap/v6/applications/" + packageName + "/items"
try:
response = requests.patch(partial_modify_item, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
Remove item
This API allows us to remove an in-app item. Here, the item ID is the required field in the request URL.
I add the item ID in the request URL and send the HTTP DELETE request. The DELETE method is used to request the server to delete a resource specified by the given URL.
itemName = "one_gallon_gas"
# Defining the API endpoint
remove_item = "https://devapi.samsungapps.com/iap/v6/applications/" + packageName + "/items/" + itemName
payload = {}
try:
response = requests.delete(remove_item, headers=headers,data=payload)
print(response.status_code)
pretty_json = json.loads(response.text)
print (json.dumps(pretty_json, indent=2))
except Exception as e:
print(str(e))
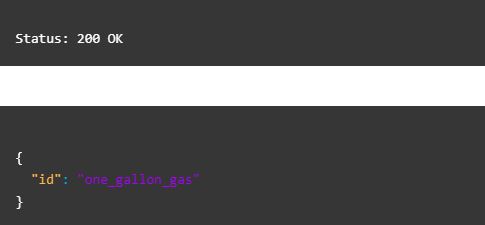
Conclusion
Other than selling paid apps, in-app purchases are the most common and popular way to monetize an app. You can integrate the Samsung In-App SDK in your app to generate profit and use the IAP Publish API to automate item-related tasks. This is the last blog article of this series. I hope you've found this article helpful. You can check out the other blogs about the Galaxy Store Developer API below.
Blog articles in this series
-
How to Create an Access Token for the Galaxy Store Developer API Using Python: Learn how to create an access token using the Galaxy Store Developer API and Python.
-
How to get statistics: Galaxy Store Statistics (GSS) is a tool that provides information about your sales, revenue, ratings, and much more, which helps you to make more effective business decisions. You can learn how to get statistical data from your apps using Python.
-
How to manage content: Manage your content in Galaxy Store by using the Content Publish API. In an app production system, managing content is a frequent task. This blog covers the implementation of this API using Python to automate your app distribution system.