Implementing Drag-and-Drop across Apps in Multi-Window Mode
Md. Iqbal Hossain
Engineer, Samsung Developer Program
One advantage of the large display in the Galaxy Z Fold series is the ability to split the screen and simultaneously use up to three apps. In multi-window mode, you can split the screen, having one window being the main focus and the other two windows being active but not focused. This means all three windows remain active, not just the largest one. You can therefore multitask in either landscape or portrait orientation, giving you even more flexibility.
The drag-and-drop feature of Android is one of the operations suitable for multi-window mode. The operation starts when the user makes a UI gesture on the application, such as a long press on a UI element, and the application recognizes it as a signal to start dragging data such as an image, video, plain text, URL, and so on. A drag shadow becomes visible during this operation to show that the event is happening. When the user releases the shadow, the operation is ended. One example of the operation is dragging a video from the file explorer to a video player application in order to play the video. The official Android guide provides a detailed article on how to implement drag-and-drop in your application.
In this blog, we show how to implement the drag-and drop-feature between multiple applications. This means we need to implement the functionality in two test applications and will also demonstrate interaction with other applications such as a web browser.
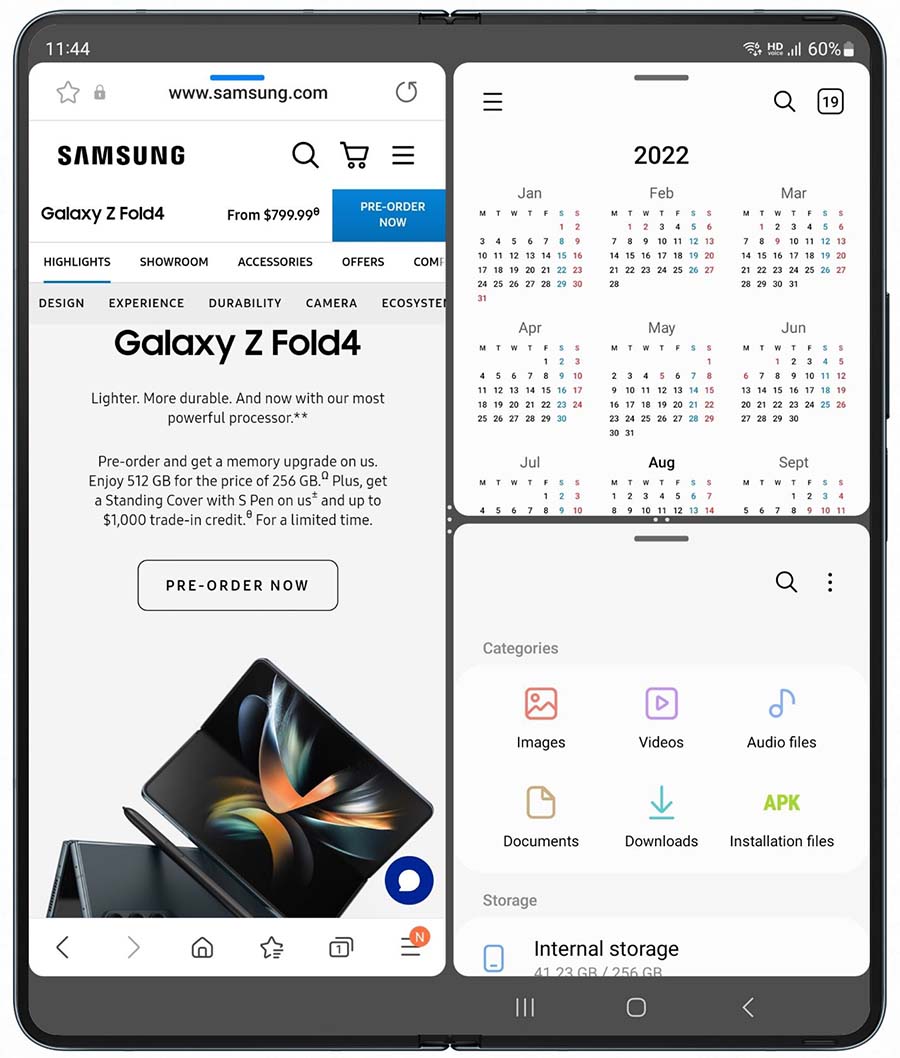
Figure 1: Multi-Window mode
So let’s see what is needed to take advantage of the multi-window mode and the drag-and-drop feature.
Implementation
Step 1
To ensure that your application works in multi-window mode, you need to add an attribute in its manifest’s <activity>
element. If you set android:resizeableActivity
to true
, the activity can be launched in multi-window or in pop-up view, and can adapt to a different screen size.
android:resizeableActivity= "true"
Step 2
To be able to drag your desired view, add setOnLongClickListener
to it, as shown below:
textview1.setOnLongClickListener { v: View ->
val item = ClipData.Item(v.tag as CharSequence)
val mimeTypes = arrayOf(ClipDescription.MIMETYPE_TEXT_PLAIN)
val data = ClipData(v.tag.toString(), mimeTypes, item)
// Instantiates the drag shadow builder
val dragshadow = View.DragShadowBuilder(v)
val flags = View.DRAG_FLAG_GLOBAL
// Starts the drag
v.startDrag(data // Data to be dragged
, dragshadow // Drag shadow builder
, v // Local data about the drag and drop operation
, flags
)
return@setOnLongClickListener true
}
DRAG_FLAG_GLOBAL
flag is required to enable drag-and-drop across applications. This means you can drop this view to another application only when the flag is set.Step 3
In MainActivity.kt
, register a drag event listener object by calling setOnDragListener
for each view of the application for which you want to enable dragging and dropping:
textview1.setOnDragListener(onDragListenerTV1)
textview2.setOnDragListener(onDragListenerTV2)
textview3.setOnDragListener(onDragListenerTV3)
Here, onDragListenerTV1
, onDragListenerTV2
, and onDragListenerTV3
are callback functions for textview1
, textview2
, and textview3
, respectively.
Step 4
Next, implement the callback functions. Here, only the callback function for textview1
is shown. You first need to declare a variable to store the action type for the incoming event.
val action: Int = event.getAction()
Step 5
Inside DragEvent.ACTION_DROP
, get the dragged item from ClipData
and check its MIME type. If the MIME type is set to text/plain
or text/html
, get the text value from the item object and allow the drop operation. Otherwise, simply show a toast message.
val item: Item = event.getClipData().getItemAt(0)
var mType = event.clipDescription.getMimeType(0)
if(mType == "text/plain" || mType== "text/html"){
// Gets the text data from the item.
dragData = item.text.toString()
}
else{
Toast.makeText(applicationContext,"Operation not allowed"+mType,Toast.LENGTH_LONG).show()
return@OnDragListener true
}
textview1
, we allow any string to be dragged and dropped. But in textview2
, only numbers are allowed and in textview3
, only email addresses are allowed. Necessary validations are added accordingly.Demonstration
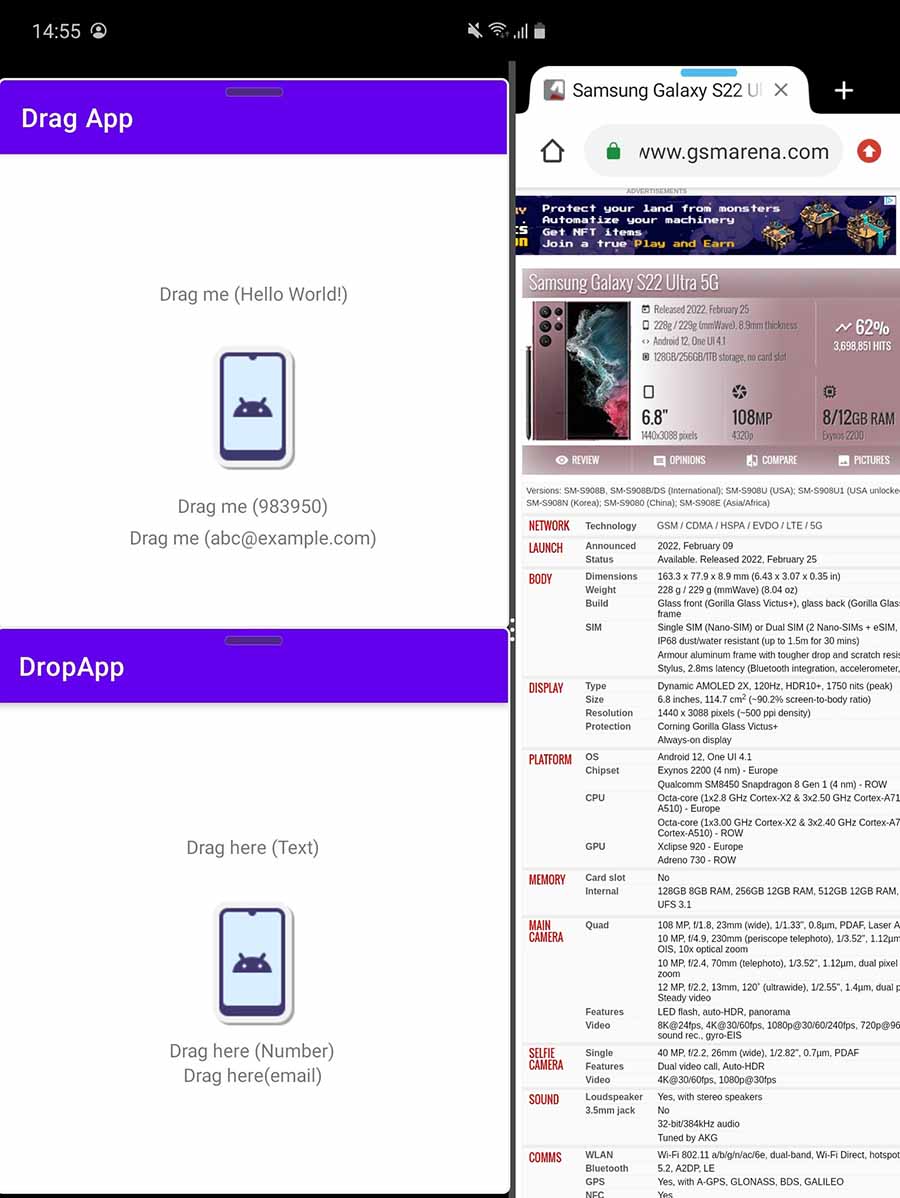
Figure 2: Both DragApp and DropApp opened in multi-window mode along with a browser
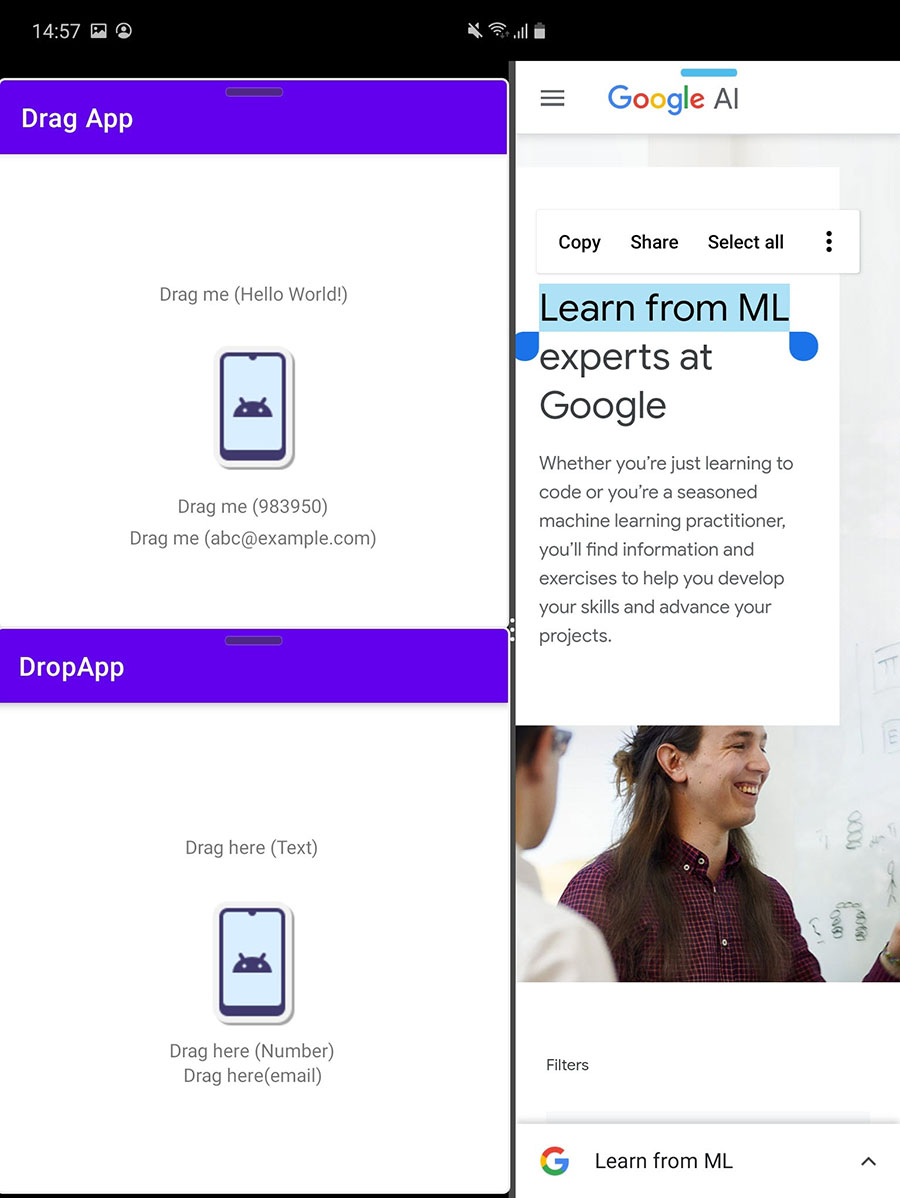
Figure 3: Selecting text from the browser
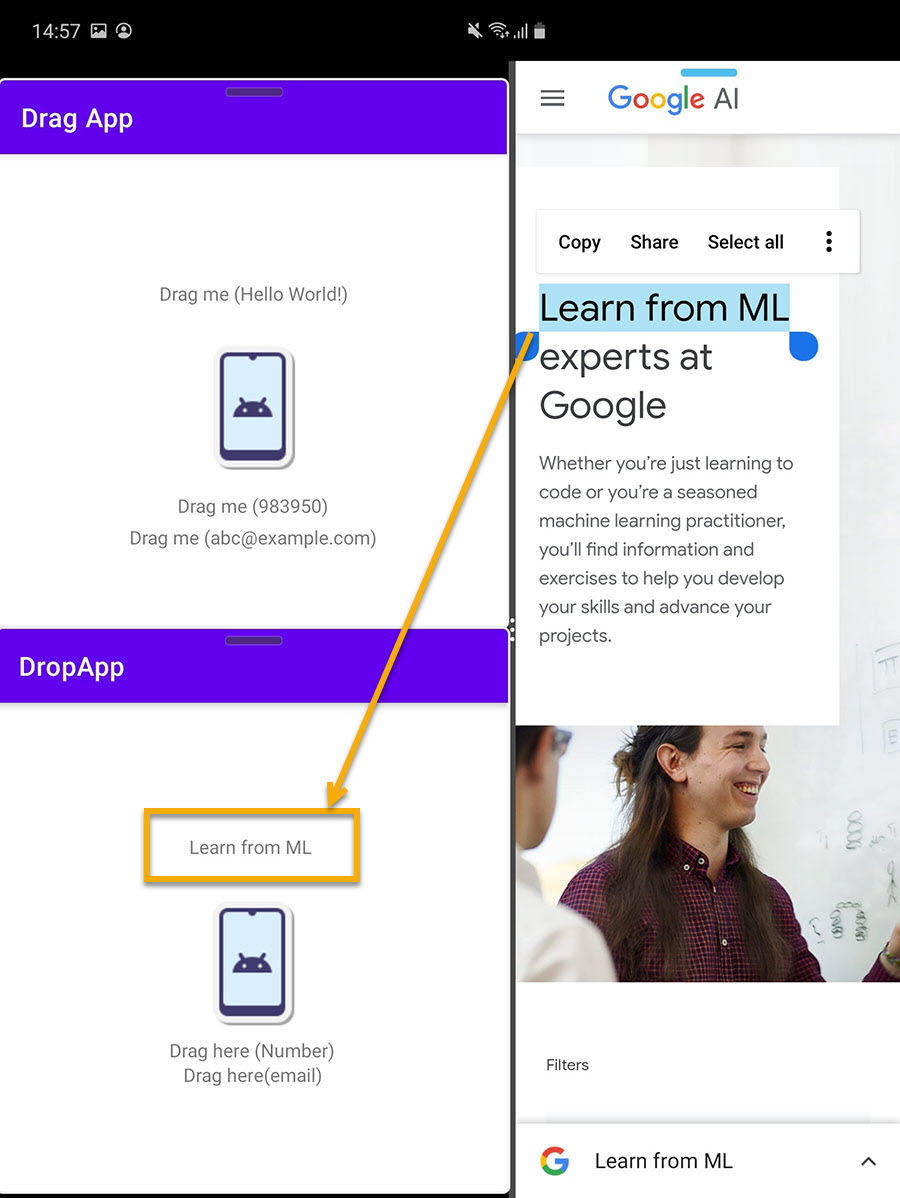
Figure 4: Text dropped into DropApp from the browser
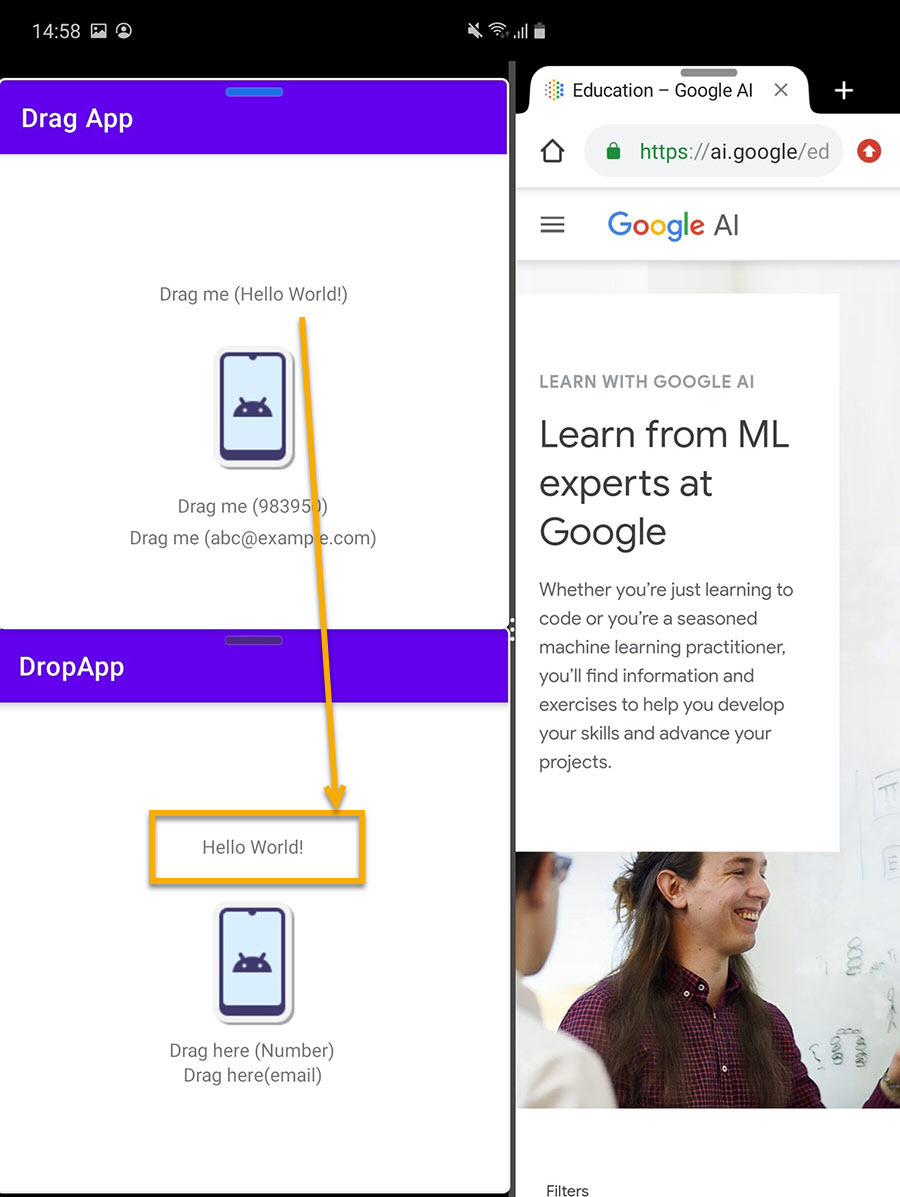
Figure 5: Text "Hello World!" dragged from DragApp to DropApp
Sample Application
A sample application has been developed to illustrate how to implement drag-and-drop between applications in multi-window mode.
Conclusion
Foldable devices provide a richer experience than phones and to take advantage of their features, every new form factor should be added to the application configuration. The drag-and-drop feature can further enhance the application user experience.
Additional resources on the Samsung Developers site
The Samsung Developers site has many resources for developers looking to build for and integrate with Samsung devices and services. Stay in touch with the latest news by creating a free account and subscribing to our monthly newsletter. Visit the Galaxy Store Games page for information on bringing your game to Galaxy Store and visit the Marketing Resources page for information on promoting and distributing your Android apps. Finally, our Developer Forum is an excellent way to stay up-to-date on all things related to the Galaxy ecosystem.