Integrate the Samsung In-App Purchase Orders API with Your Application
Md. Hossain
Engineer, Samsung Developer Program
Samsung In-App Purchase (IAP) offers developers a robust solution for handling digital transactions within mobile applications available on Galaxy Store. Whether it is selling digital goods, handling subscriptions, or managing refunds, Samsung IAP is designed to offer a smooth, secure experience. The Samsung IAP Orders API expands the scope of these benefits. You can fetch all the payments and refunds history according to specified dates. This content guides you through the essential components for implementing both the Samsung IAP and Samsung IAP Orders APIs.
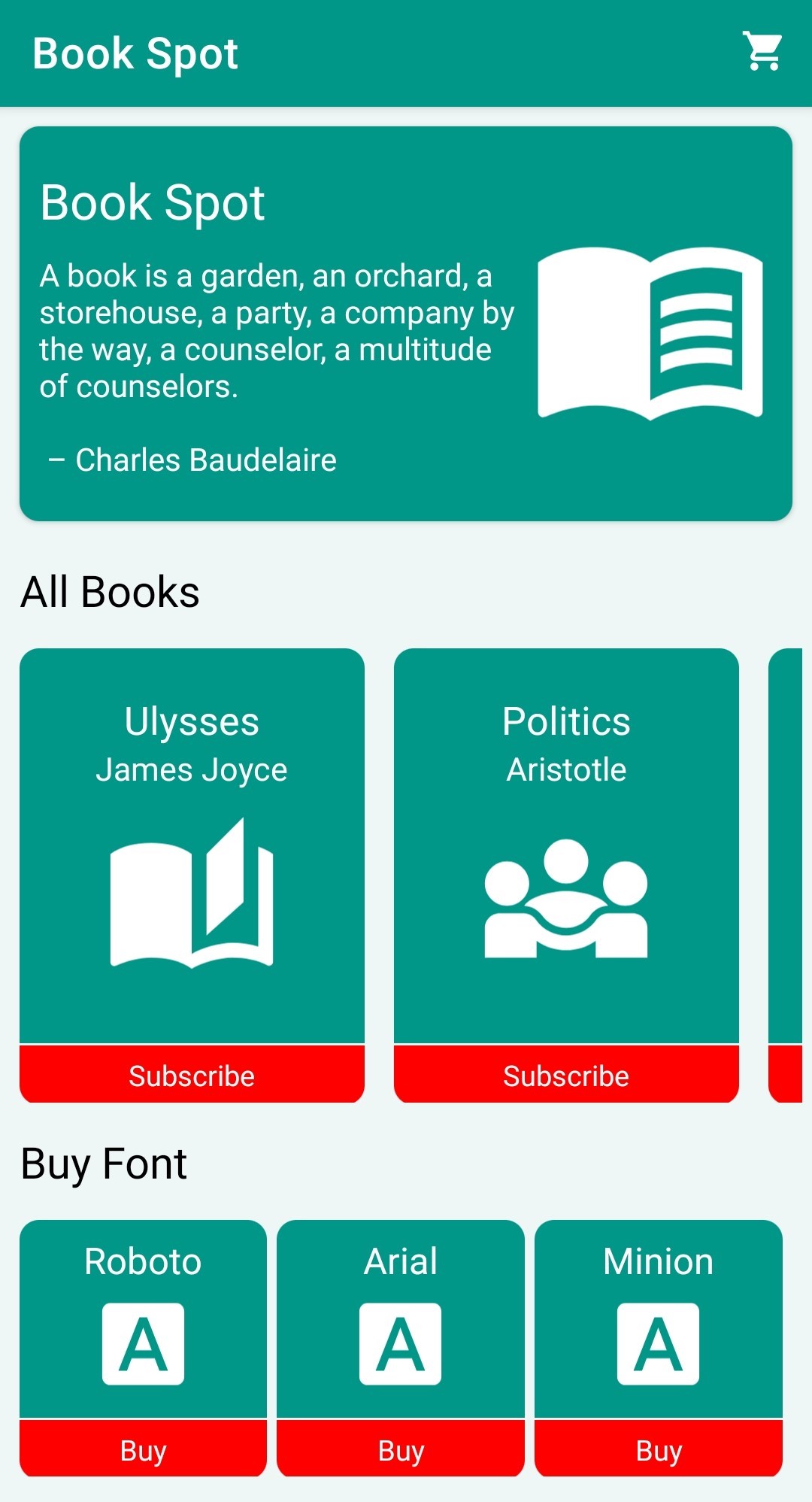
In this tutorial, we provide a sample application called Book Spot, which offers users the option to subscribe to their favorite books and consumable items, such as text fonts, for purchase. After purchase, users can consume the item. Finally, developers can view all their payment and refund history on specific dates by calling the Samsung IAP Orders API from the back-end server.
Prerequisites
Before implementing in-app purchases in your app, do the following to enable a smooth and effective execution of the process while developing your own application:
- Integrate the Samsung IAP SDK into your application. For more information about the IAP SDK integration, you can follow the Integration of Samsung IAP Services in Android Apps article.
- Upload the application for Beta testing on Samsung Galaxy Store. A step-by-step guide with screenshots has been provided in the documentation. For more details, see the section “Production Closed Beta Test” on the Test Guide.
- Finally, create items on the Seller Portal so that users can purchase or subscribe to them while using the application. For more details about the available items that the Seller Portal supports, see the Programming Guide.
For the sample application, we have already completed these steps. Some example items were already created in Seller Portal, such as books and fonts so that you can consume and subscribe to them while using this sample application.
Implementation of Item Purchase
Now that the application and items are ready, you can implement the purchase functionality in your application like in the sample below:
- When clicking "Buy," the
startPayment()
method is called, specifying parameters for item ID and theOnPaymentListener
interface, which handles the results of the payment transaction. - The
onPayment()
callback returns whether the purchase has succeeded or failed. ThepurchaseVo
object is instantiated and in case it is not null, it holds the purchase results. - If the purchase is successful, then it validates the purchase showing its ID. If the purchase is not successful, a purchaseError message is shown. For more information, check the Purchase an in-app item section.
iapHelper.startPayment(itemId, String.valueOf(1), new OnPaymentListener() {
@Override
public void onPayment(@NonNull ErrorVo errorVo, @Nullable PurchaseVo purchaseVo) {
if (purchaseVo != null) {
// Purchase Successful
Log.d("purchaseId" , purchaseVo.getPurchaseId().toString());
Toast.makeText(getApplicationContext() ,"Purchase Successfully",
Toast.LENGTH_SHORT).show();
} else {
Log.d("purchaseError" , errorVo.toString());
Toast.makeText(getApplicationContext() ,"Purchase Failed",
Toast.LENGTH_SHORT).show();
}
}
});
Implementation of Item Consumption
After successfully purchasing an item, the user can then consume it. In the sample code below, when "Consumed" is selected, the consumePurchaseItems()
triggers the consume functionality. This is necessary as items must be marked as consumed so they can be purchased again:
- The
consumePurchaseItems()
method is called specifying the parameters forpurchaseId
and theOnConsumePurchasedItemsListener()
interface, which handles the item data and results. - This code also checks if consuming the purchased items succeeded or failed:
- If the
errorVo
parameter is not null and there is no error with the purchase, which can be verified with theIAP_ERROR_NONE
response code, then the “Purchase Acknowledged” message is displayed. - However, if there is an error, the
errorVo
parameter returns an error description with thegetErrorString()
getter, along with the “Acknowledgment Failed” message.
- If the
iapHelper.consumePurchasedItems(purchaseId, new OnConsumePurchasedItemsListener() {
@Override
public void onConsumePurchasedItems(@NonNull ErrorVo errorVo, @NonNull
ArrayList<ConsumeVo>arrayList) {
if (errorVo != null && errorVo.getErrorCode() == iapHelper.IAP_ERROR_NONE) {
Toast.makeText(getApplicationContext() ,"Purchase Acknowledged",
Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(getApplicationContext(), "Acknowledgment Failed: " +
errorVo.getErrorString(), Toast.LENGTH_SHORT).show();
}
}
});
Implementation of Item Subscription
Besides purchasing and consuming items, you can also subscribe to them in your applications. Similar to the validation done for the consumable item purchase, you validate the subscription with a purchase ID if the purchase is successful. Use the same code snippet specified for “Item Purchase.” For more information, check the Implementation of Item Purchase section.
Implementation of the Samsung IAP Orders API
The Samsung IAP Orders API is used to view all payments and refunds on a specific date. It does this by fetching the payments and refunds history within the date you specified. Let’s implement the Samsung IAP Orders API and create a server to listen to its notifications. Through server-to-server communication, the API returns all orders data for the application.
Configuring the Server
You can develop a Spring Boot server for this purpose. Here are the guidelines on how to set up this server:
- Set up a Spring Boot Project. For more information, follow the steps on Developing Your First Spring Boot Application.
- Set up your server endpoint:
-
Create a controller for the Samsung IAP Orders API in an integrated development environment (IDE) after importing the Spring Boot project you created. This helps managing all in-app order-related activities and processing them within your application.
-
The controller receives
POST
requests sent from Samsung’s IAP orders service ensuring the communication with your application.
-
Get Payment and Refund History
To view all payments and refunds:
- You must make a
POST
request to the Samsung IAP Orders API endpoint with the required headers specified below. - If you specify a date, all the payment history for this date is returned. Otherwise, it only returns all the data from the day before the current date.
API Endpoint: https://devapi.samsungapps.com/iap/seller/orders
Method: POST
Headers:
Add the following fields to the request header. For more information, see the Create an Access Token page, which helps you understand how to create the access token in detail. The token is used for authorization. You can also get the Service Account ID
by clicking the Assistance > API Service tabs on Seller Portal. For more details, read the section Create a service account and visit Seller Portal.
Header Name | Description | Required/Optional | Values |
---|---|---|---|
Content-Type | Format of the request body | Required | application/json |
Authorization | Authorization security header | Required | Bearer: access_token |
Service Account ID | This ID can be created in Seller Portal and is used to generate the JSON Web Token (JWT) | Required | service-account-id |
Parameters:
The following parameters can be used to build your POST
request.
Name | Type | Required/Optional | Description |
---|---|---|---|
sellerSeq | String | Required | Your seller deeplink, which is found in your profile in Seller Portal and consists of a 12-digit number. |
packageName | String | Optional | Used to view payment and refund data. You can provide the application package name. When a package name is not specified, the data for all applications is shown. |
requestDate | String | Optional | Specify a date from which to view the payment and refund data. If the date is not specified, the data from a day before your current date is returned. |
continuationToken | String | Optional | Use this if you want to check if there is a continuation for the data on the next page. If there is no more data, the response is null. |
To implement REST API support, add the following OkHttp
library dependencies to your application's build.gradle
file:
implementation 'com.squareup.okhttp3:okhttp: version'
implementation 'com.google.code.gson:gson: version'
A detailed description of the request items can be found in the Request section of the Samsung IAP Orders API documentation. For more information on the server communication, see Samsung IAP Server API. Here is a brief summary of the code below:
- A
POST
request is mapped to the/orders
URL, which logs the request. - The previously described parameters containing the data you specified are formatted in a JSON body using the
String.format()
method. - The outgoing request is logged in a
JSON
body format. - A
RequestBody
is instantiated containing theJSON data
, formatted for an HTTP request to be sent to the server with the specified token and Service Account ID. - This code also handles multiple results your request can return:
- The
onFailure()
method is called when the network request fails for some reason, providing any error details using theIOException
exception. - If the request succeeds, the
onResponse()
method returns the response body or any response exception found.
- The
@RestController
@RequestMapping(value = "/iap", method = RequestMethod.POST)
public class OrdersController {
private final OkHttpClient client = new OkHttpClient();
@GetMapping("/orders")
public void sendToServer() {
System.out.println("POST request received"); // Log the request
// Define parameters values, use according to your requirement
// String packageName = "com.example.app_name ";
// String requestDate = "20240615";
// String continuationToken = "XXXXXXXXXXX…….XXXXXX";
String sellerSeq = "0000000XXXXX";
// Create the JSON body, use packageName, requestDate, continuationToken according to your requirement
String jsonBody = String.format(
"{\"sellerSeq\":\"%s\"}",
sellerSeq
);
// Create the request body
RequestBody body = RequestBody.create(jsonBody, MediaType.parse("application/json; charset=utf-8"));
// Access token
String token = "0DjT9yzrYUKDoGbVUlXXXXXX";
// Build the request
Request request = new Request.Builder()
.url("https://devapi.samsungapps.com/iap/seller/orders")
.post(body)
.addHeader("Authorization","Bearer " + token)
.addHeader("service-account-id", "85412253-21b2-4d84-8ff5-XXXXXXXXXXXX")
.addHeader("content-type", "application/json")
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(@NotNull Call call, @NotNull IOException e) {
System.err.println("Request failed: " + e.getMessage());
}
@Override
public void onResponse(@NotNull Call call, @NotNull Response response) throws IOException {
if (response.isSuccessful()) {
String responseBody = response.body().string();
System.out.println("Response: " + responseBody);
} else {
System.err.println("Unexpected response code: " + response.code());
System.err.println("Response body: " + response.body().string());
}
response.close(); // Close the response body
}
});
}
}
Congratulations! You have just built the Spring Boot server to handle API POST requests using the OkHttpClient to manage HTTP requests and responses for your sample application.
Example Response
As previously mentioned, a JSON-formatted response is returned to your request. For detailed descriptions of each response body element, see the “Response” section of the Samsung IAP Orders API documentation. The following output format is a sample in which only some of the response-body data is presented.
- In this case, the
continuationToken
parameter key returns null because there is no continuation for the data on the next page. - The
orderItemList
parameter key lists all the orders with specific details, such asorderId
,countryId
,packageName
, among others.
{
"continuationToken": null,
"orderItemList": [
{
"orderId": "S20230210KR019XXXXX",
"purchaseId": "a778b928b32ed0871958e8bcfb757e54f0bc894fa8df7dd8dbb553cxxxxxxxx",
"contentId": "000005059XXX",
"countryId": "USA",
"packageName": "com.abc.xyz"
},
{
"orderId": "S20230210KR019XXXXX",
"purchaseId": "90a5df78f7815623eb34f567eb0413fb0209bb04dad1367d7877edxxxxxxxx",
"contentId": "000005059XXX",
"countryId": "USA",
"packageName": "com.abc.xyz"
},
]
}
Usually, the responses contain all the relevant information about user purchases, such as the in-app item title, price, and payment status. Therefore, you can use the information and create views for an easier order management.
Conclusion
You have learned how to implement item purchase, consumption, and registration, as well as how to integrate the Samsung IAP Orders API and configure a server to fetch all the payment and refund history within specific dates.
Integrating the Samsung IAP Orders API functionality into your server is an essential step in managing your application payments history to ensure a seamless experience to users. Now, you can implement the Samsung IAP Orders API into your application to track all payments, refunds and make your business more manageable.
Related Resources
For additional information on this topic, see the resources below: