Integrate a Payment UI into Your TRON DApp
M.A. Hasan Molla
Engineer, Samsung Developer Program
The Samsung Blockchain Platform (SBP) SDK offers a full set of functionalities for developing decentralized applications (DApps) that communicate with the Ethereum and TRON networks. The SDK includes a simple payment UI that you can integrate into your DApp, enabling users to easily make TRX, TRC-10, and TRC-20 transactions.
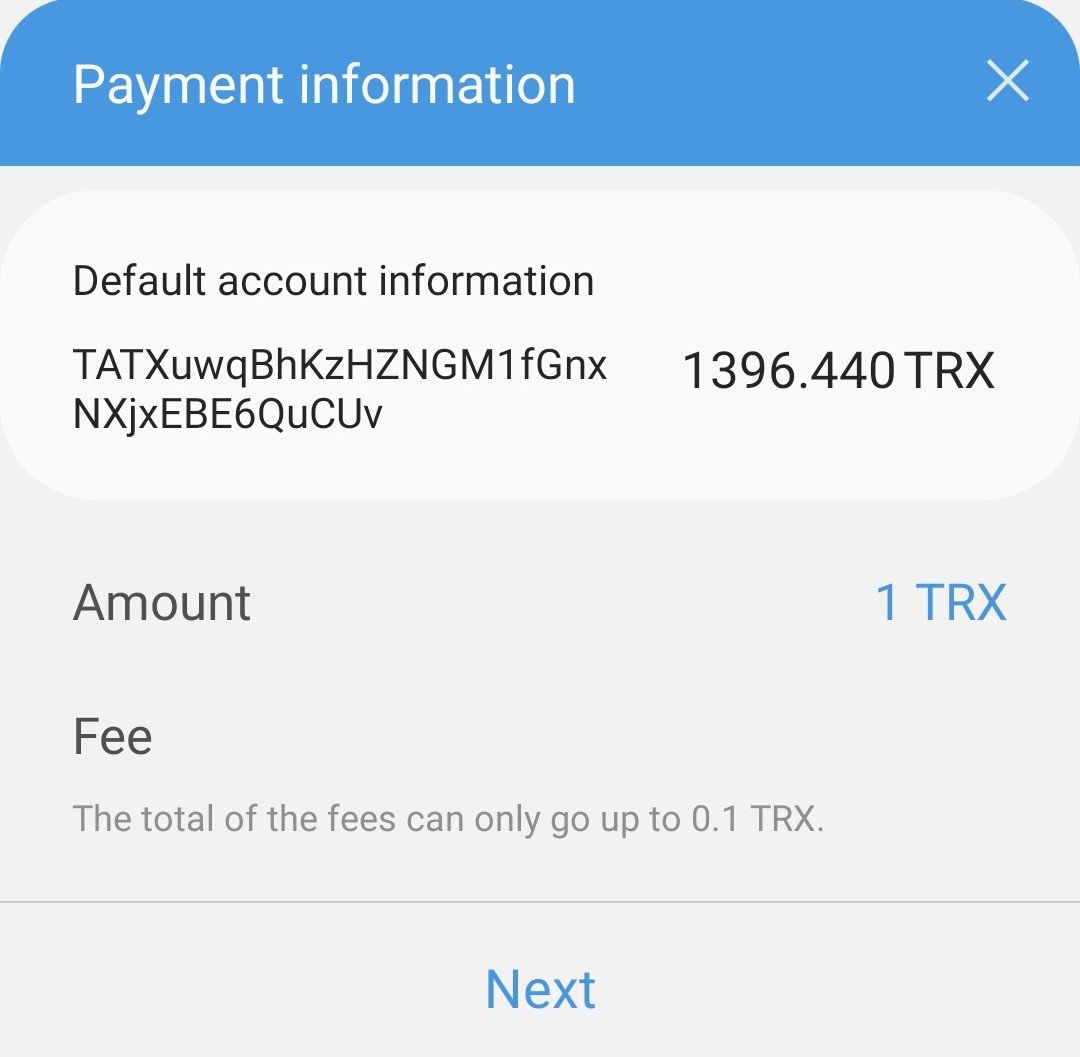
Figure 1: SBP SDK payment sheet
This article describes a sample application that demonstrates how to integrate the SBP SDK payment UI into a TRON DApp. You can follow along with the demonstration by downloading the sample application.
The application connects to a hardware wallet, creates a new account, and fetches the account balance. Then, it launches the payment sheet UI to make a transaction. Each of these actions has been implemented with a corresponding button to help you understand the sequence of steps and visualize the result.
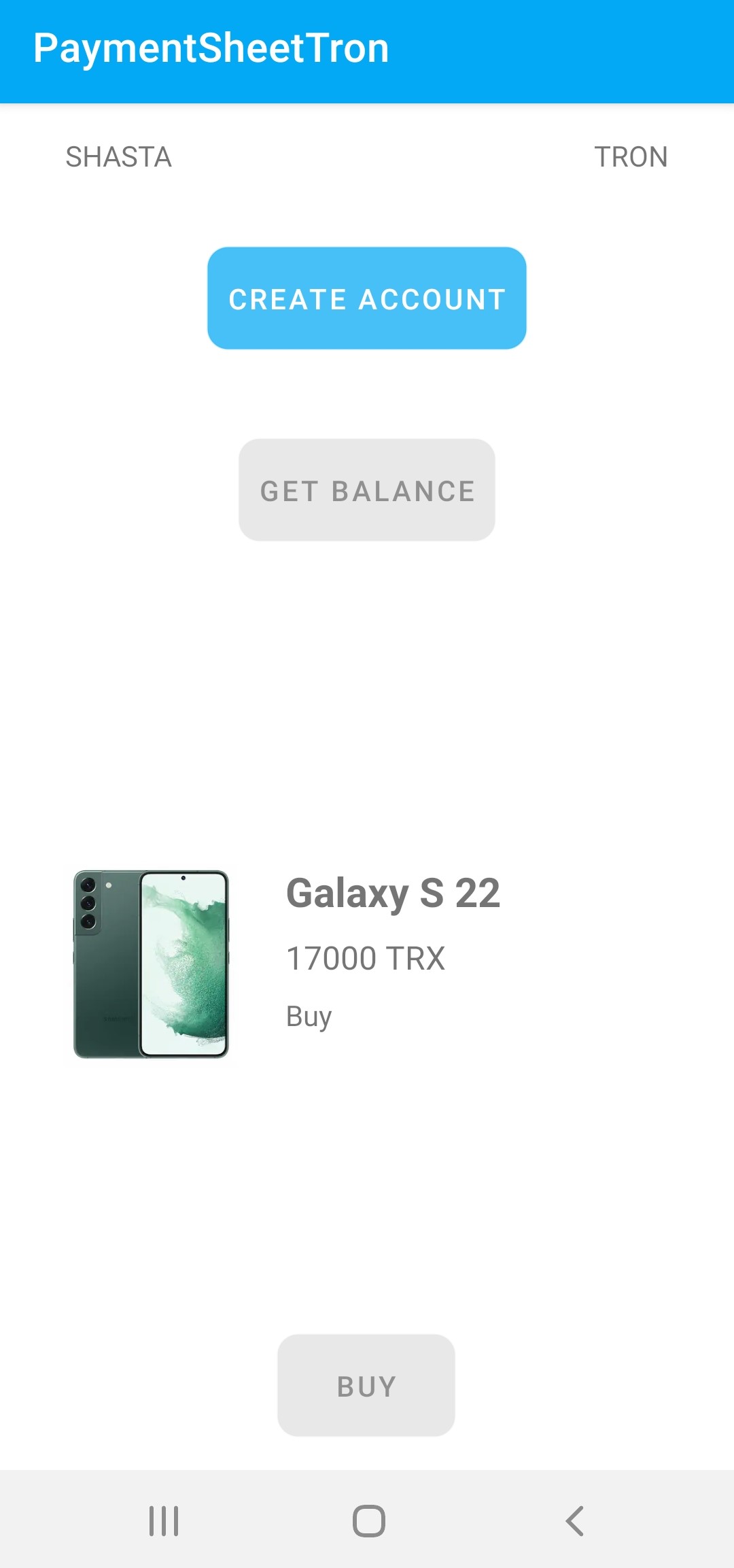
Figure 2: Sample application overview
Initialize the SBP SDK instance
To communicate with the SBP SDK, you must create an instance of the SBlockchain class.
Before creating the instance, implement the SBP SDK dependencies and set up your device environment to use the Samsung Blockchain Keystore as a hardware wallet. For detailed information about the dependencies and the environment settings, see Getting Started.
To create the SBlockchain class instance, you can use the following code:
try {
SBlockchain mSblockchain = new SBlockchain();
mSblockchain.initialize(context);
} catch (SsdkUnsupportedException e) {
if (e.getErrorType() == VENDOR_NOT_SUPPORTED){
Log.e("error", "Platform SDK does not support this device");
}
}
Connect to a hardware wallet
To manage accounts and create transactions, you must connect to a hardware wallet. The SBP SDK supports specific hardware wallets, such as the Samsung Blockchain Keystore and Ledger Nano.
The sample application supports the Samsung Blockchain Keystore, which is integrated into selected Samsung devices. It is secured with the Samsung Knox TrustZone and the defense-grade Trusted Execution Environment (TEE). To learn more about the security features, see Samsung Blockchain Keystore SDK Overview.
Use the connect()
method to connect with the hardware wallet. To specify Samsung Blockchain Keystore as the hardware wallet, set the hardware wallet type to HardwareWalletType.SAMSUNG
. For more information, see Connect With Hardware Wallet.
HardwareWalletType mHardwareWalletType = HardwareWalletType.SAMSUNG;
HardwareWalletManager mHardwareWalletManager = mSblockchain.getHardwareWalletManager();
mHardwareWalletManager.connect(mHardwareWalletType, true)
.setCallback(new ListenableFutureTask.Callback<HardwareWallet>() {
@Override
public void onSuccess(HardwareWallet hardwareWallet) {
mHardwareWallet = hardwareWallet;
Log.i(LOG_TAG, "Wallet connection successful.");
}
@Override
public void onFailure(@NotNull ExecutionException e) {
Log.e(LOG_TAG, "Wallet connection failed.");
}
@Override
public void onCancelled(@NotNull InterruptedException e) {
Log.e(LOG_TAG, "Wallet connection cancelled.");
}
});
In the sample application, when the wallet is connected, its Wallet ID is displayed.
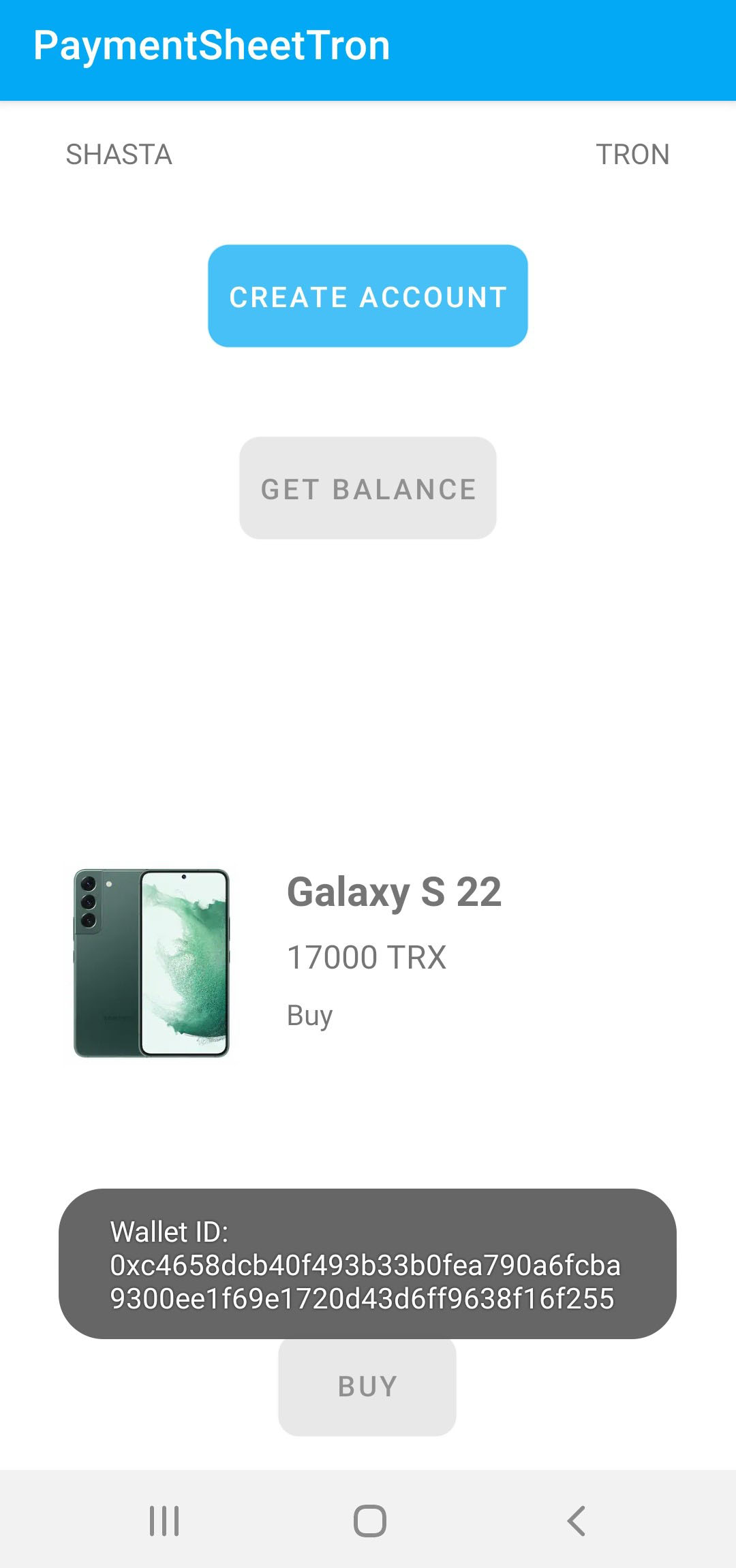
Figure 3: Hardware wallet connected
Manage accounts
The SBP SDK includes various account management features, such as generating, restoring, and retrieving accounts.
To transfer TRX, you must have a TRON account. A TRON account includes various attributes, such as the TRX balance, bandwidth, and energy. For more information about the TRON account model, see the official Tron documentation.
The following code snippet shows how to retrieve TRON accounts from the wallet:
CoinType coinType = CoinType.TRX;
NetworkType networkType = TronNetworkType.SHASTA;
String rpc = "https://api.shasta.trongrid.io";
mCoinNetworkInfo = new CoinNetworkInfo(coinType, networkType, rpc);
private List<Account> accountList = mSblockchain.getAccountManager()
.getAccounts(mHardwareWallet.getWalletId(), mCoinNetworkInfo.getCoinType(), mCoinNetworkInfo.getNetworkType());
To create a TRON account, use the generateNewAcount()
method:
mAccountManager.generateNewAccount(mHardwareWallet, mCoinNetworkInfo)
.setCallback(new ListenableFutureTask.Callback<Account>() {
@Override
public void onSuccess(Account account) {
Log.i(LOG_TAG, "Account created.");
}
@Override
public void onFailure(@NotNull ExecutionException e) {
Log.e(LOG_TAG, "Account creation failed.");
Log.e(LOG_TAG, "" + e.getMessage());
}
@Override
public void onCancelled(@NotNull InterruptedException e) {
Log.e(LOG_TAG, "Account creation cancelled.");
}
});
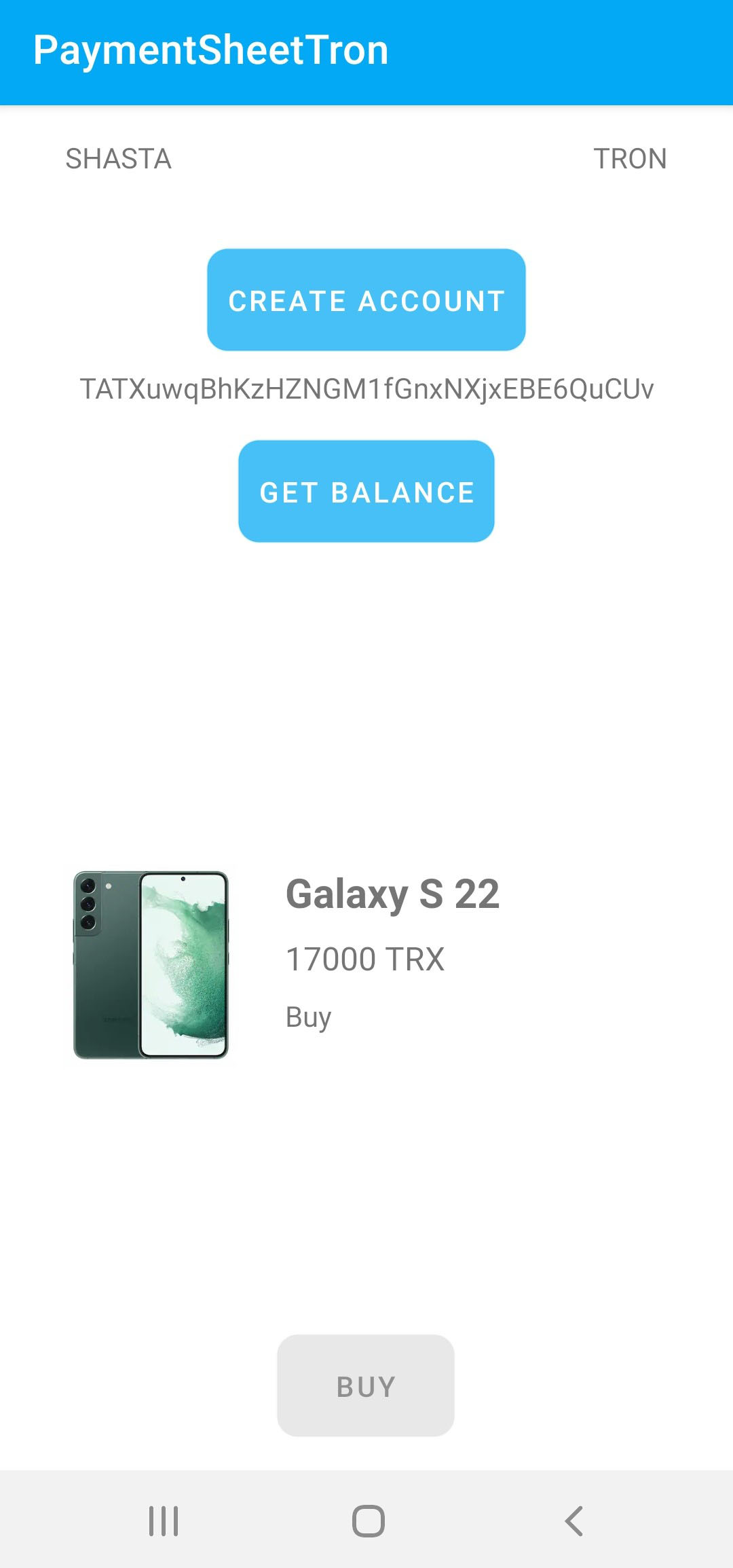
Figure 4: Account retrieved
Fetching the account balance
For the purpose of this demonstration, get some TRX for the SHASTA TRON test network from the SHASTA faucet.
To retrieve balance information for your TRON account from the network, use the getBalance()
method:
CoinService mCoinService = CoinServiceFactory.getCoinService(getApplicationContext(), mCoinNetworkInfo);
TronService tronService = (TronService) mCoinService;
tronService.getBalance(firstAccount).setCallback(new ListenableFutureTask.Callback<BigInteger>() {
@Override
public void onSuccess(BigInteger bigInteger) {
amountInTrx = TronUtils.convertSunToTrx(bigInteger);
Log.i(LOG_TAG, "Balance fetched successfully.");
Log.i(LOG_TAG, "Balance is:" + amountInTrx.toString());
}
@Override
public void onFailure(@NotNull ExecutionException e) {
Log.e(LOG_TAG, "Balance fetching failed.");
Log.e(LOG_TAG, "" + e.getMessage());
}
@Override
public void onCancelled(@NotNull InterruptedException e) {
Log.e(LOG_TAG, "Balance fetching cancelled.");
}
});
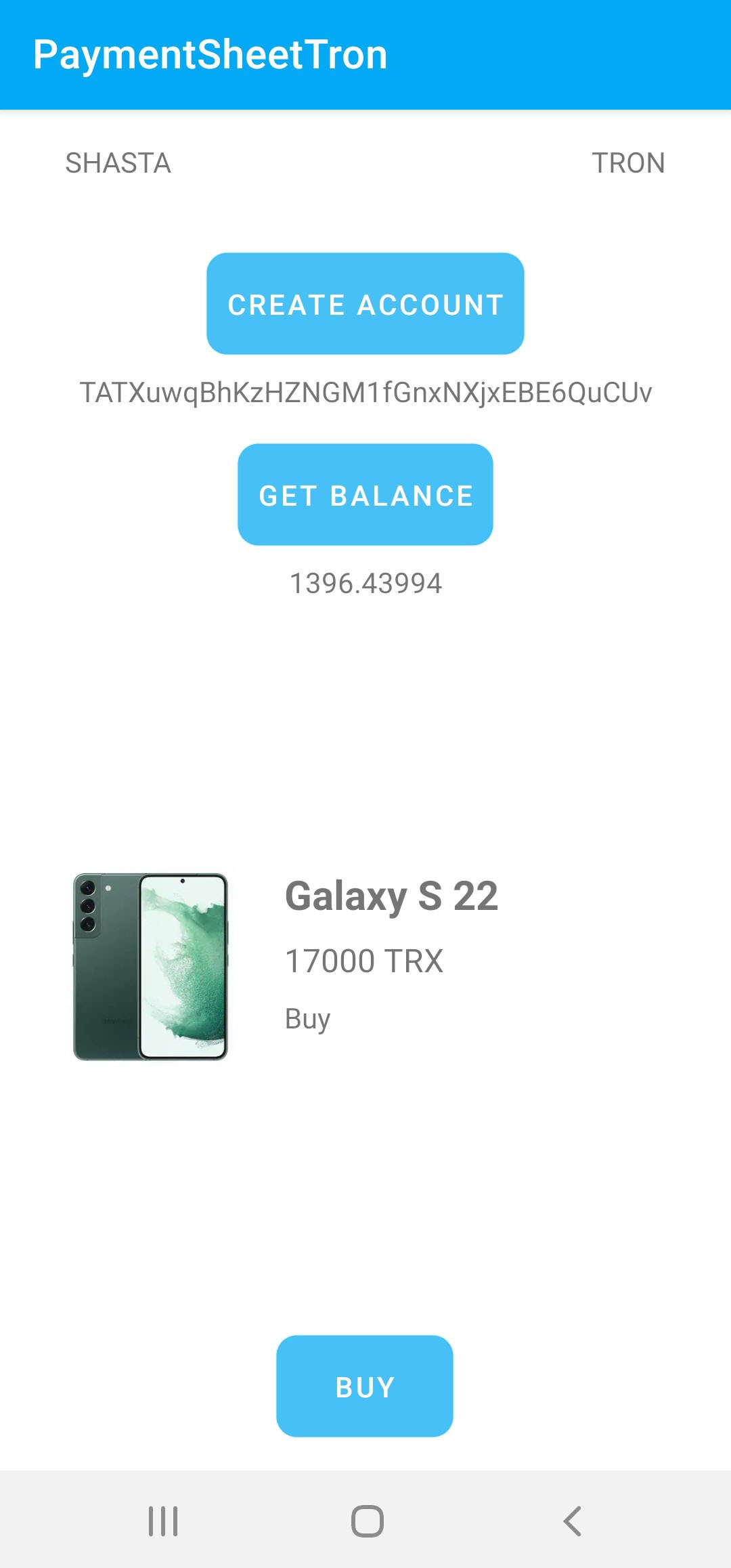
Figure 5: Account balance retrieved
Launch the payment sheet intent
The simple payment UI included in the SBP SDK shows all the information you need for the transaction, such as the destination, amount, and fees.
The createTronPaymentSheetActivityIntent()
method creates an intent of the TRON payment sheet activity for TRX transactions:
String toAddress = "TMie1hFH6zgxHVNnDWkVNRpHHitGuM7mjN";
BigDecimal sendAmount = new BigDecimal("1.0");
BigInteger convertedSendAmount = TronUtils.convertTrxToSun(sendAmount);
try {
Intent intent = tronService.createTronPaymentSheetActivityIntent(
this,
mHardwareWallet,
(TronAccount) firstAccount,
toAddress,
convertedSendAmount
);
activityResultLauncher.launch(intent);
} catch (Exception e) {
Log.e(LOG_TAG, "Error in sending: " + e);
}
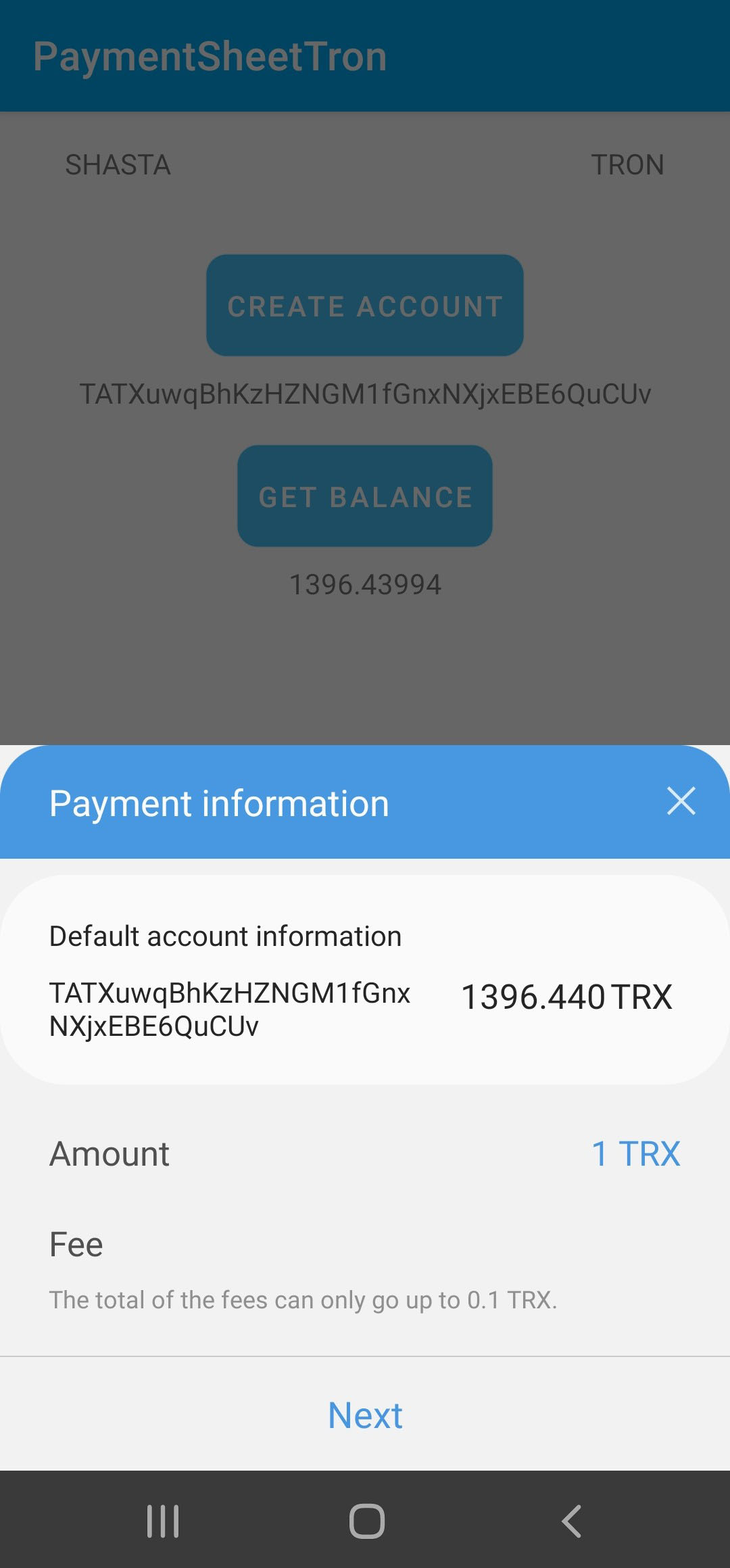
Figure 6: Payment sheet launched
You can also use the createTrc10PaymentSheetActivityIntent()
and createTrc20PaymentSheetActivityIntent()
methods to create intents for TRC-10 and TRC-20 token payment sheet activities, respectively. A token ID is required to create a TRC-10 payment and a token address is required to create a TRC-20 payment.
Get the transaction result
You can retrieve the transaction result using the ActivityResultLauncher
class. To get the transaction hash in the received intent parameter, use the txid key. To get the error for a failed transaction, use the error key.
ActivityResultLauncher<Intent> activityResultLauncher = registerForActivityResult(
new ActivityResultContracts.StartActivityForResult(),
new ActivityResultCallback<ActivityResult>() {
@Override
public void onActivityResult(ActivityResult result) {
switch (result.getResultCode()) {
case Activity.RESULT_OK:
Log.d(LOG_TAG, "Transaction Hash: " + result.getData().getStringExtra("txid"));
break;
case Activity.RESULT_CANCELED:
Log.e(LOG_TAG, "Transaction Failed: " + result.getData().getStringExtra("error"));
break;
}
}
}
);
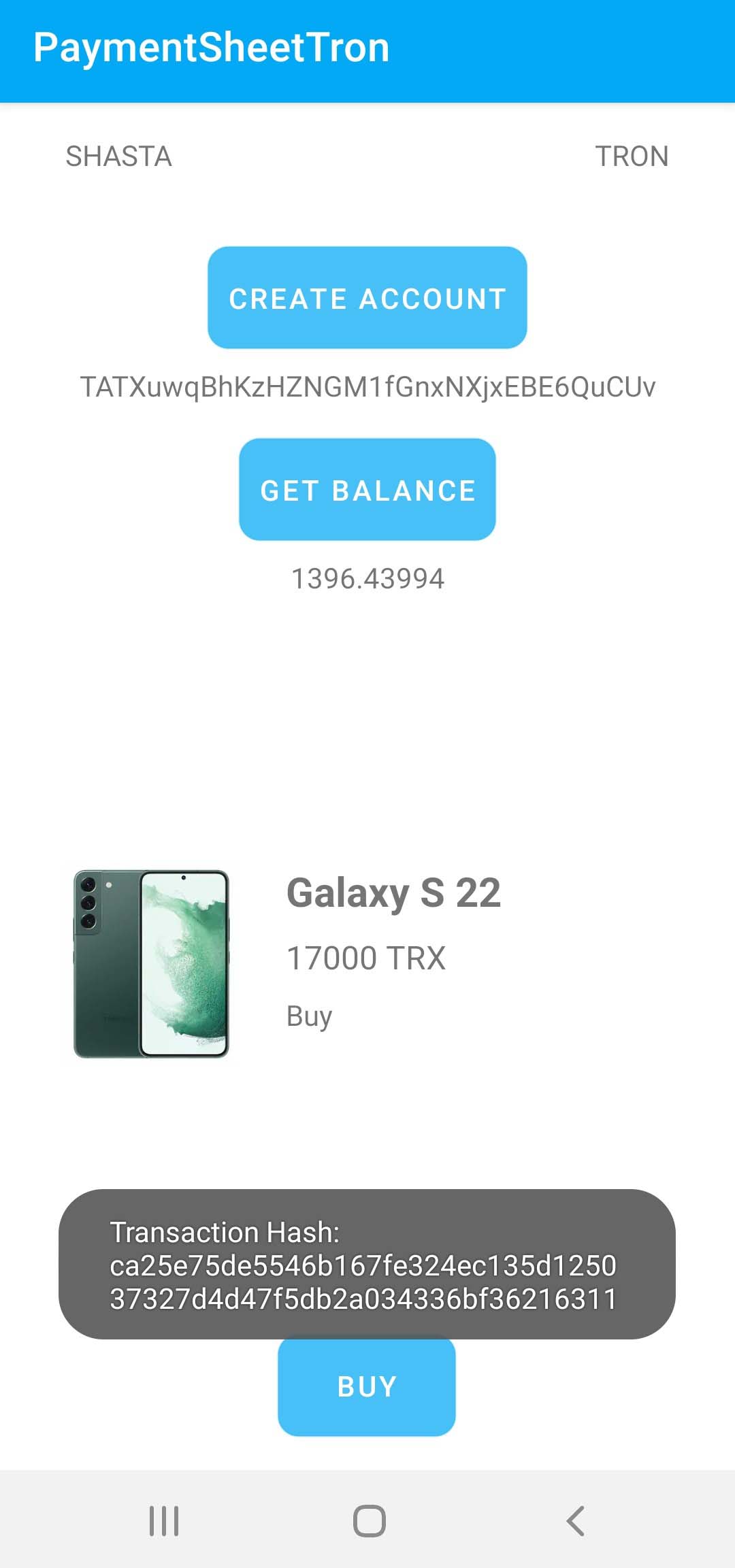
Figure 7: Transaction hash retrieved
This article has demonstrated how you can implement the TRON payment sheet UI provided by the SBP SDK in your DApp. The payment sheet UI simplifies your application development and gives your users a consistent user experience.
Additional resources
- Download the payment sheet sample application
- Simple Payment UI programming guide
- Samsung Blockchain Platform SDK overview
- Samsung Blockchain Platform SDK sample application
Related articles
- Send TRX with the Samsung Blockchain Platform SDK
- Take Your TRON DApp to Galaxy Devices Around the World
Additional resources on the Samsung Developers site
The Samsung Developers site has many resources for developers looking to build for and integrate with Samsung devices and services. Stay in touch with the latest news by creating a free account and subscribing to our monthly newsletter. Visit the Galaxy Store Games page for information on bringing your game to Galaxy Store and visit the Marketing Resources page for information on promoting and distributing your Android apps. Finally, our Developer Forum is an excellent way to stay up-to-date on all things related to the Galaxy ecosystem.